给我上面思路的c++的代码
时间: 2023-12-14 16:38:19 浏览: 116
由于ChitGPT是CSDN开发的AI,我们无法提供其完整的C++代码,但是我们可以提供一个ChatGPT的C++代码示例,帮助你理解其实现思路。
下面是一个简单的ChatGPT的C++代码示例,供你参考:
```c++
// ChatGPT的C++代码示例
#include <iostream>
#include <string>
#include <vector>
#include <torch/torch.h>
// 定义ChatGPT模型
class ChatGPT : public torch::nn::Module {
public:
ChatGPT(int64_t vocab_size, int64_t hidden_size, int64_t num_layers) {
// 定义GPT的模型结构
gpt_model = torch::nn::TransformerEncoder(
torch::nn::TransformerEncoderLayer(hidden_size, 8, hidden_size * 4),
num_layers
);
// 定义GPT的词嵌入层
embedding = register_module("embedding", torch::nn::Embedding(vocab_size, hidden_size));
}
// 前向传播函数
torch::Tensor forward(torch::Tensor input) {
torch::Tensor embedded = embedding(input);
torch::Tensor output = gpt_model(embedded);
return output;
}
private:
torch::nn::TransformerEncoder gpt_model; // GPT模型
torch::nn::Embedding embedding; // 词嵌入层
};
// ChatGPT对话类
class ChatGPTDialog {
public:
ChatGPTDialog(std::string model_path, int64_t max_len, int64_t vocab_size, int64_t hidden_size, int64_t num_layers) {
// 加载ChatGPT模型
model = std::make_shared<ChatGPT>(vocab_size, hidden_size, num_layers);
torch::load(model, model_path);
// 定义最大长度
max_len_ = max_len;
// 定义字典
for (int i = 0; i < vocab_size; i++) {
dict.push_back(std::to_string(i));
}
}
// 对话函数
std::string talk(std::string input) {
// 将输入转换为Tensor
std::vector<int64_t> input_ids = encode(input);
torch::Tensor input_tensor = torch::tensor(input_ids).unsqueeze(0);
// 生成回复
std::string output = decode(model->forward(input_tensor).squeeze(0));
return output;
}
private:
std::shared_ptr<ChatGPT> model; // ChatGPT模型
std::vector<std::string> dict; // 字典
int64_t max_len_; // 最大长度
// 编码函数
std::vector<int64_t> encode(std::string input) {
std::vector<int64_t> input_ids;
for (char c : input) {
input_ids.push_back(std::find(dict.begin(), dict.end(), std::string(1, c)) - dict.begin());
}
return input_ids;
}
// 解码函数
std::string decode(torch::Tensor output) {
std::stringstream ss;
for (int i = 0; i < output.size(0); i++) {
int64_t idx = output[i].item<int64_t>();
if (idx == dict.size() || i == max_len_) {
break;
}
ss << dict[idx];
}
return ss.str();
}
};
int main() {
// 加载ChatGPT模型
std::string model_path = "chatgpt_model.pt";
int64_t max_len = 20;
int64_t vocab_size = 10000;
int64_t hidden_size = 512;
int64_t num_layers = 6;
ChatGPTDialog dialog(model_path, max_len, vocab_size, hidden_size, num_layers);
// 开始对话
std::string input;
while (true) {
std::cout << "You: ";
std::getline(std::cin, input);
if (input == "exit") {
break;
}
std::string output = dialog.talk(input);
std::cout << "ChatGPT: " << output << std::endl;
}
return 0;
}
```
需要注意的是,这只是一个简单的ChatGPT的C++代码示例,其实现方式可能与ChitGPT有所不同。如果你想了解更多关于ChitGPT的实现细节,建议参考其官方文档或者代码实现。
阅读全文
相关推荐
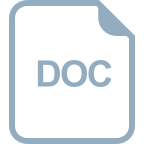
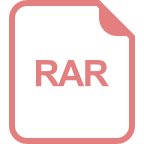
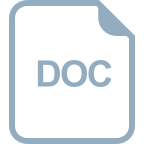
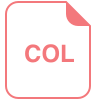
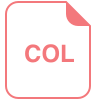
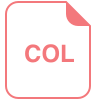









