C语言AES128算法实现
时间: 2023-10-30 21:07:56 浏览: 96
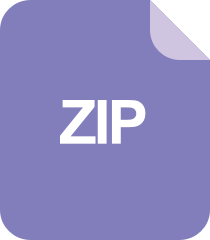
AES算法的C语言实现

由于AES128算法的实现比较复杂,需要用到很多底层的加密算法,因此需要一些专业的加密库来实现。常用的加密库有OpenSSL、GnuTLS、BouncyCastle等。
以下是使用OpenSSL库实现AES128加密的示例代码:
```c
#include <openssl/aes.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
unsigned char *key = (unsigned char *)"0123456789abcdef";
unsigned char *plaintext = (unsigned char *)"hello world!";
unsigned char iv[AES_BLOCK_SIZE];
memset(iv, 0x00, AES_BLOCK_SIZE);
AES_KEY aes_key;
if (AES_set_encrypt_key(key, 128, &aes_key) < 0) {
fprintf(stderr, "AES_set_encrypt_key error\n");
return -1;
}
int len = strlen((char *)plaintext);
unsigned char *ciphertext = (unsigned char *)malloc(len + AES_BLOCK_SIZE);
memset(ciphertext, 0x00, len + AES_BLOCK_SIZE);
AES_cbc_encrypt(plaintext, ciphertext, len, &aes_key, iv, AES_ENCRYPT);
printf("plaintext: %s\n", plaintext);
printf("ciphertext: ");
for (int i = 0; i < len + AES_BLOCK_SIZE; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
free(ciphertext);
return 0;
}
```
以上代码中,使用AES_set_encrypt_key()函数设置密钥,使用AES_cbc_encrypt()函数进行加密。其中,iv为初始化向量,用于增强加密强度。
需要注意的是,AES加密算法是对称加密算法,因此加密和解密使用的密钥是相同的。在实际使用中,需要注意密钥的保护和管理,避免密钥被泄露。
阅读全文
相关推荐
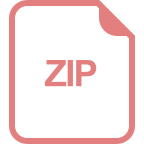
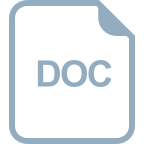
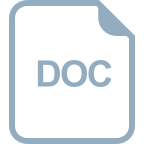
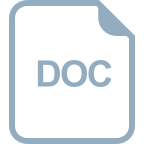

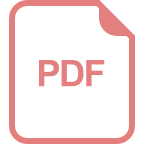
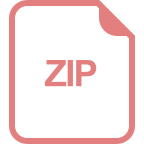
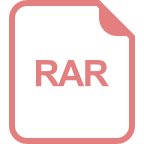
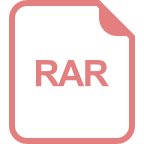
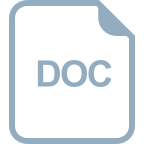
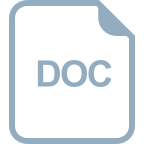
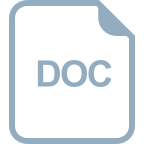
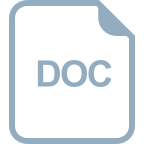
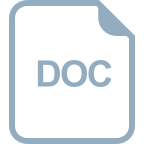
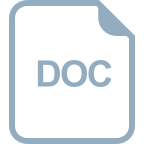
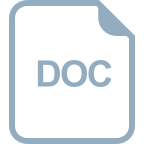