基于openssl库实现C语言AES128算法代码
时间: 2024-10-27 13:09:07 浏览: 44
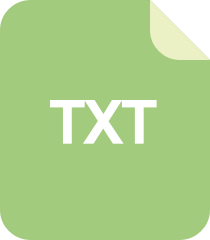
AES源代码(完全用C语言实现)

在C语言中使用OpenSSL库实现AES128加密通常涉及以下几个步骤:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
#include <openssl/evp.h>
// AES128 key and IV (16 bytes each)
const unsigned char aes_key[] = "0123456789abcdef01234567";
const unsigned char aes_iv[] = "fedcba9876543210";
void aes_encrypt(unsigned char *plaintext, int plaintext_len,
unsigned char *key, unsigned char *iv) {
AES_KEY aes;
AES_set_encrypt_key(key, 128, &aes); // 128 is for AES-128
unsigned char ciphertext[plaintext_len + AES_BLOCK_SIZE]; // Room for padding
int ciphertext_len;
int res = AES_cbc_encrypt(plaintext, ciphertext, plaintext_len,
&aes, iv, AES_ENCRYPT);
if (res == 0) {
ciphertext_len = plaintext_len; // No padding in this example
} else {
// Handle encryption error or padding here
ciphertext_len = -1;
}
// Output encrypted data (or store it securely)
if (ciphertext_len >= 0) {
printf("Encrypted:\n");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x ", ciphertext[i]);
}
printf("\n");
}
}
int main() {
const char* plaintext = "Hello, world!";
int plaintext_len = strlen(plaintext);
aes_encrypt((unsigned char*)plaintext, plaintext_len, aes_key, aes_iv);
return 0;
}
```
这个例子展示了如何使用AES-128-CBC模式对明文进行加密,但请注意,实际应用中你需要处理加密错误、添加正确的PKCS#7填充等细节。
阅读全文
相关推荐
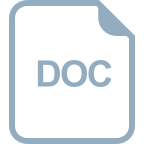
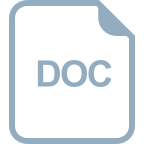

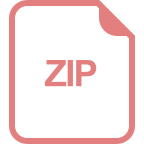
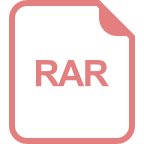
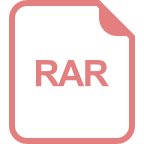
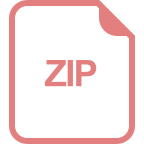
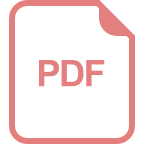
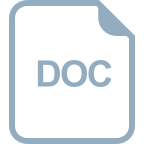
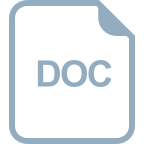







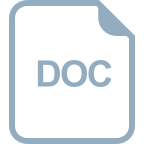