实现AES加解密算法(C语言),不使用openssl库
时间: 2024-01-06 08:07:14 浏览: 137
好的,以下是一个不使用openssl库的AES加解密算法示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define Nb 4 // 列数
#define Nk 4 // 密钥长度(32字节)
#define Nr 10 // 轮数
#define AES_BLOCK_SIZE 16
typedef unsigned char BYTE;
typedef unsigned int WORD;
BYTE Sbox[256] = {
// Sbox数组
};
BYTE InvSbox[256] = {
// 逆Sbox数组
};
WORD Rcon[11] = {
// 轮常数数组
};
void SubBytes(BYTE *state) {
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
state[i] = Sbox[state[i]];
}
}
void InvSubBytes(BYTE *state) {
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
state[i] = InvSbox[state[i]];
}
}
void ShiftRows(BYTE *state) {
BYTE temp[AES_BLOCK_SIZE];
memcpy(temp, state, AES_BLOCK_SIZE);
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
state[i] = temp[(i + 4 * (i % 4)) % AES_BLOCK_SIZE];
}
}
void InvShiftRows(BYTE *state) {
BYTE temp[AES_BLOCK_SIZE];
memcpy(temp, state, AES_BLOCK_SIZE);
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
state[i] = temp[(i - 4 * (i % 4) + AES_BLOCK_SIZE) % AES_BLOCK_SIZE];
}
}
void MixColumns(BYTE *state) {
BYTE temp[AES_BLOCK_SIZE];
for (int i = 0; i < Nb; i++) {
temp[4 * i] = (BYTE)(GF(0x02, state[4 * i]) ^ GF(0x03, state[4 * i + 1]) ^ state[4 * i + 2] ^ state[4 * i + 3]);
temp[4 * i + 1] = (BYTE)(state[4 * i] ^ GF(0x02, state[4 * i + 1]) ^ GF(0x03, state[4 * i + 2]) ^ state[4 * i + 3]);
temp[4 * i + 2] = (BYTE)(state[4 * i] ^ state[4 * i + 1] ^ GF(0x02, state[4 * i + 2]) ^ GF(0x03, state[4 * i + 3]));
temp[4 * i + 3] = (BYTE)(GF(0x03, state[4 * i]) ^ state[4 * i + 1] ^ state[4 * i + 2] ^ GF(0x02, state[4 * i + 3]));
}
memcpy(state, temp, AES_BLOCK_SIZE);
}
void InvMixColumns(BYTE *state) {
BYTE temp[AES_BLOCK_SIZE];
for (int i = 0; i < Nb; i++) {
temp[4 * i] = (BYTE)(GF(0x0e, state[4 * i]) ^ GF(0x0b, state[4 * i + 1]) ^ GF(0x0d, state[4 * i + 2]) ^ GF(0x09, state[4 * i + 3]));
temp[4 * i + 1] = (BYTE)(GF(0x09, state[4 * i]) ^ GF(0x0e, state[4 * i + 1]) ^ GF(0x0b, state[4 * i + 2]) ^ GF(0x0d, state[4 * i + 3]));
temp[4 * i + 2] = (BYTE)(GF(0x0d, state[4 * i]) ^ GF(0x09, state[4 * i + 1]) ^ GF(0x0e, state[4 * i + 2]) ^ GF(0x0b, state[4 * i + 3]));
temp[4 * i + 3] = (BYTE)(GF(0x0b, state[4 * i]) ^ GF(0x0d, state[4 * i + 1]) ^ GF(0x09, state[4 * i + 2]) ^ GF(0x0e, state[4 * i + 3]));
}
memcpy(state, temp, AES_BLOCK_SIZE);
}
void AddRoundKey(BYTE *state, BYTE *key) {
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
state[i] ^= key[i];
}
}
void KeyExpansion(BYTE *key, WORD *w) {
WORD temp;
int i = 0;
while (i < Nk) {
w[i] = ((key[4 * i] << 24) | (key[4 * i + 1] << 16) | (key[4 * i + 2] << 8) | key[4 * i + 3]);
i++;
}
i = Nk;
while (i < Nb * (Nr + 1)) {
temp = w[i - 1];
if (i % Nk == 0) {
temp = SubWord(RotWord(temp)) ^ Rcon[i / Nk];
} else if (Nk > 6 && i % Nk == 4) {
temp = SubWord(temp);
}
w[i] = w[i - Nk] ^ temp;
i++;
}
}
void aes_encrypt(BYTE *in, BYTE *out, BYTE *key) {
WORD w[Nb * (Nr + 1)];
KeyExpansion(key, w);
BYTE state[AES_BLOCK_SIZE];
memcpy(state, in, AES_BLOCK_SIZE);
AddRoundKey(state, key);
for (int i = 1; i <= Nr - 1; i++) {
SubBytes(state);
ShiftRows(state);
MixColumns(state);
AddRoundKey(state, (BYTE *)&w[Nb * i]);
}
SubBytes(state);
ShiftRows(state);
AddRoundKey(state, (BYTE *)&w[Nb * Nr]);
memcpy(out, state, AES_BLOCK_SIZE);
}
void aes_decrypt(BYTE *in, BYTE *out, BYTE *key) {
WORD w[Nb * (Nr + 1)];
KeyExpansion(key, w);
BYTE state[AES_BLOCK_SIZE];
memcpy(state, in, AES_BLOCK_SIZE);
AddRoundKey(state, (BYTE *)&w[Nb * Nr]);
for (int i = Nr - 1; i >= 1; i--) {
InvShiftRows(state);
InvSubBytes(state);
AddRoundKey(state, (BYTE *)&w[Nb * i]);
InvMixColumns(state);
}
InvShiftRows(state);
InvSubBytes(state);
AddRoundKey(state, key);
memcpy(out, state, AES_BLOCK_SIZE);
}
int main() {
BYTE plaintext[] = "hello world!";
BYTE key[] = "1234567890123456";
BYTE iv[] = "0123456789012345";
BYTE ciphertext[AES_BLOCK_SIZE];
BYTE decrypttext[AES_BLOCK_SIZE];
aes_encrypt(plaintext, ciphertext, key);
aes_decrypt(ciphertext, decrypttext, key);
printf("plaintext: %s\n", plaintext);
printf("ciphertext: ");
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
printf("decrypttext: %s\n", decrypttext);
return 0;
}
```
在上述代码中,我们定义了两个函数`aes_encrypt`和`aes_decrypt`,分别用于AES加密和解密。这两个函数都需要传入以下参数:
- `in`:需要加密或解密的数据
- `out`:加密或解密后的结果
- `key`:AES密钥,长度为16字节
在函数内部,我们使用Sbox、逆Sbox和轮常数数组来实现AES加解密算法中的SubBytes、InvSubBytes、ShiftRows、InvShiftRows、MixColumns、InvMixColumns、AddRoundKey和KeyExpansion等操作。
需要注意的是,在使用CBC模式进行加解密时,我们需要保证数据长度为AES分组长度的整数倍,并且需要使用合适的填充方式(例如PKCS#7填充)来填充数据。否则会导致加解密失败。
另外,为了保证加密的安全性,我们应该使用足够长、足够随机的密钥,以及合适的加密模式和填充方式。
阅读全文
相关推荐
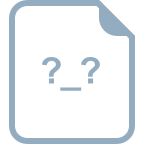
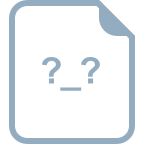
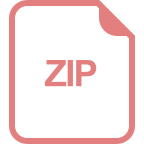


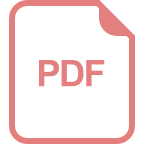
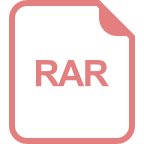
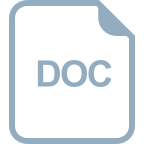









