128位密钥的aes加解密算法c语言
时间: 2023-07-27 10:02:42 浏览: 253
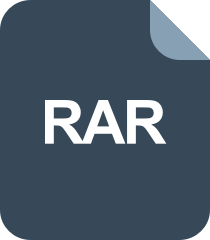
C语言实现AES128加解密算法
AES(Advanced Encryption Standard)是一种对称加密算法,使用相同的密钥进行加密和解密。128位密钥的AES算法是其最常用的变体之一。
以下是一个使用C语言实现128位密钥AES加解密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <string.h>
#include <openssl/aes.h>
void aes_encrypt(const uint8_t* key, const uint8_t* plaintext, uint8_t* ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_encrypt(plaintext, ciphertext, &aes_key);
}
void aes_decrypt(const uint8_t* key, const uint8_t* ciphertext, uint8_t* plaintext) {
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_decrypt(ciphertext, plaintext, &aes_key);
}
int main() {
const uint8_t key[] = "0123456789abcdef"; // 128位密钥
const uint8_t plaintext[] = "Hello, AES!";
int plaintext_len = strlen((char*)plaintext);
uint8_t ciphertext[plaintext_len];
uint8_t decryptedtext[plaintext_len];
aes_encrypt(key, plaintext, ciphertext);
aes_decrypt(key, ciphertext, decryptedtext);
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext (hex): ");
for(int i = 0; i < plaintext_len; ++i) {
printf("%02x ", ciphertext[i]);
}
printf("\n");
printf("Decrypted text: %s\n", decryptedtext);
return 0;
}
```
以上代码使用OpenSSL库中的AES函数实现了对128位密钥的AES加解密操作。首先定义了`aes_encrypt`和`aes_decrypt`两个函数来执行加密和解密,然后在`main`函数中定义了要加密的明文和密钥。通过调用`aes_encrypt`函数进行加密,然后再调用`aes_decrypt`函数进行解密,并打印结果。
请注意,这是一个简单的示例代码,实际使用中需要进行适当的错误处理、填充操作等。另外,确保在使用密钥时,采取适当的方式来生成和保存密钥,以保证安全性。
阅读全文
相关推荐
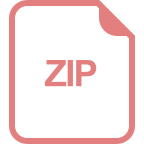
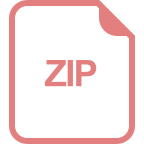
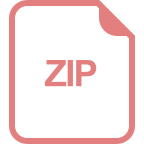

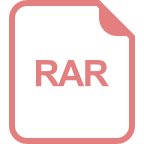
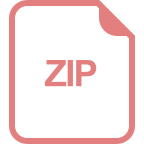
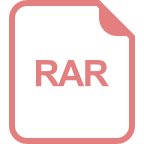
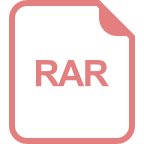
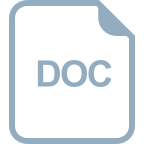
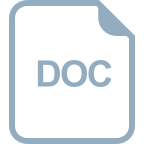






