// // Created by NLER on 2023/5/24. // #include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdbool.h> #define MAX_SIZE 100 typedef char DataType; typedef struct stack{ DataType data[MAX_SIZE]; int length; }*Stack; Stack init_Stack(){ Stack stack = (Stack) malloc(sizeof (Stack)); stack -> length = -1; } void push(DataType e,Stack stack){ if(stack -> length != MAX_SIZE){ stack -> data[stack -> length] = e; stack -> length++; } else{ printf("data full"); } } void pop(DataType *e,Stack stack){ if(stack -> length == -1) { printf("data empty\n"); } else{ stack -> length--; e = stack -> data[stack -> length]; } } bool is_empty(Stack stack){ if(stack -> length == -1){ return true; } else return false; } DataType get_top(Stack stack){ return stack -> data[stack -> length]; } int get_prior(char c){ if(c == '+' || c == '-'){ return 1; } else if(c == '/' || c == ''){ return 2; } else if(c == '('){ return 0; } } int main(){ Stack stack = init_Stack(); char buf[1024]; scanf("%s",buf); // 中缀转后缀 for(int i = 0 ; i < strlen(buf); i++){ // printf("input char is %c\n",buf[i]); if(buf[i] >= '0' && buf[i] <= '9'){ printf("%c",buf[i]); } if(buf[i] == '('){ push(buf[i],stack); } else if(buf[i] == ')'){ if(!is_empty(stack)){ // 不是空的,那就看看顶部元素是不是( while (get_top(stack) != '(' || is_empty(stack)){ char top = ' '; pop(&top,stack); printf("%c",top); } if(get_top(stack) == '('){ char top = ' '; pop(&top,stack); } } } else{ // 是算数运算符 if(is_empty(stack)){ push(buf[i],stack); } else{ if(get_prior(buf[i]) > get_prior(get_top(stack))){ push(buf[i], stack); } else{ // 把不符合条件的给派出去 while(get_prior(buf[i]) <= get_prior(get_top(stack)) || !is_empty(stack)){ char top = ' '; pop(&top,stack); printf("%c",top); } // 然后开始插入关键的数据 push(buf[i],stack); } } } } }修改这段代码,实现中缀表达式转后缀表达式
时间: 2024-01-10 22:04:44 浏览: 25
这段代码中有一些错误和不完整的地方,我来帮你修改一下,实现中缀表达式转后缀表达式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#define MAX_SIZE 100
typedef char DataType;
typedef struct stack{
DataType data[MAX_SIZE];
int length;
} *Stack;
Stack init_Stack(){
Stack stack = (Stack) malloc(sizeof(struct stack));
stack->length = -1;
return stack;
}
void push(DataType e, Stack stack){
if(stack->length != MAX_SIZE - 1){
stack->length++;
stack->data[stack->length] = e;
}
else{
printf("stack full\n");
}
}
void pop(DataType *e, Stack stack){
if(stack->length == -1){
printf("stack empty\n");
}
else{
*e = stack->data[stack->length];
stack->length--;
}
}
bool is_empty(Stack stack){
if(stack->length == -1){
return true;
}
else{
return false;
}
}
DataType get_top(Stack stack){
if(stack->length != -1){
return stack->data[stack->length];
}
else{
printf("stack empty\n");
return ' ';
}
}
int get_prior(char c){
if(c == '+' || c == '-'){
return 1;
}
else if(c == '*' || c == '/'){
return 2;
}
else if(c == '('){
return 0;
}
else{
return -1; // 如果是非法字符,返回 -1
}
}
void infix_to_postfix(char* infix, char* postfix){
Stack stack = init_Stack();
int i = 0, j = 0;
while(infix[i] != '\0'){
if(infix[i] >= '0' && infix[i] <= '9'){ // 如果是数字,直接输出到后缀表达式中
postfix[j] = infix[i];
j++;
}
else if(infix[i] == '('){ // 如果是左括号,入栈
push(infix[i], stack);
}
else if(infix[i] == ')'){ // 如果是右括号,依次弹出栈顶元素并输出,直到遇到左括号
while(get_top(stack) != '(' && !is_empty(stack)){
DataType top = ' ';
pop(&top, stack);
postfix[j] = top;
j++;
}
if(get_top(stack) == '('){ // 左括号出栈
DataType top = ' ';
pop(&top, stack);
}
else{ // 括号不匹配,输出错误信息
printf("parentheses not match\n");
return;
}
}
else{ // 如果是运算符,弹出栈顶元素并输出,直到遇到优先级不高于当前运算符的元素,然后将当前运算符入栈
while(!is_empty(stack) && get_prior(infix[i]) <= get_prior(get_top(stack))){
DataType top = ' ';
pop(&top, stack);
postfix[j] = top;
j++;
}
push(infix[i], stack);
}
i++;
}
// 如果中缀表达式遍历完毕,但栈中还有元素,依次弹出栈顶元素并输出
while(!is_empty(stack)){
DataType top = ' ';
pop(&top, stack);
postfix[j] = top;
j++;
}
postfix[j] = '\0';
}
int main(){
char infix[1024], postfix[1024];
printf("input infix expression: ");
scanf("%s", infix);
infix_to_postfix(infix, postfix);
printf("postfix expression: %s\n", postfix);
return 0;
}
```
这里主要修改了以下几个问题:
1. 在 `init_Stack` 函数中,分配内存时应该使用 `sizeof(struct stack)` 而不是 `sizeof(Stack)`,因为 `Stack` 只是一个指针类型,大小为 8 个字节(64 位系统),而结构体 `stack` 的大小为 104 个字节(64 位系统),两者是不同的。
2. 在 `push` 函数中,应该先把 `length` 加 1,然后再将元素入栈。
3. 在 `pop` 函数中,应该传入一个指针类型的参数,用于返回弹出的元素。
4. 在 `get_top` 函数中,如果栈为空,应该输出错误信息,并返回一个字符 ' '。
5. 在 `get_prior` 函数中,如果是非法字符,应该返回 -1。
6. 在 `infix_to_postfix` 函数中,需要判断括号是否匹配,如果不匹配,输出错误信息并返回。
7. 在 `infix_to_postfix` 函数中,最后需要把后缀表达式的最后一个字符设为 '\0',以表示字符串结束。
其他的小问题也做了一些修改,代码应该可以正常工作了。
相关推荐
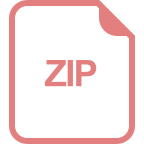
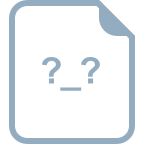
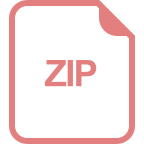




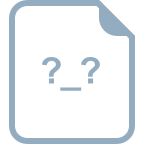
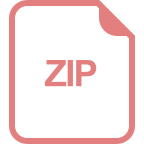
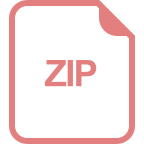
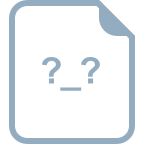
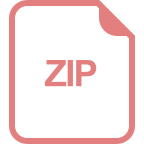
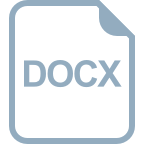
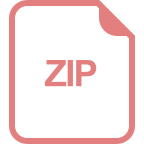