java 模拟ATM机编写一个Account类模拟账户,创建一个有10个账户的数组,定义Account类的一个子类Newaccount,包括name存储名字
时间: 2023-08-09 10:04:14 浏览: 40
以及balance存储余额,并实现以下功能:
1. 存款:deposit(double amount)
2. 取款:withdraw(double amount)
3. 查询余额:getBalance()
4. 转账:transfer(Newaccount other, double amount)
Account类的代码如下:
```java
public class Account {
private int id;
private double balance;
public Account(int id, double balance) {
this.id = id;
this.balance = balance;
}
public void deposit(double amount) {
balance += amount;
System.out.println("Deposit successful! Your balance is now " + balance);
}
public void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
System.out.println("Withdrawal successful! Your balance is now " + balance);
} else {
System.out.println("Insufficient balance!");
}
}
public double getBalance() {
return balance;
}
public void transfer(Newaccount other, double amount) {
if (balance >= amount) {
balance -= amount;
other.deposit(amount);
System.out.println("Transfer successful! Your balance is now " + balance);
} else {
System.out.println("Insufficient balance!");
}
}
}
```
Newaccount类的代码如下:
```java
public class Newaccount extends Account {
private String name;
public Newaccount(int id, double balance, String name) {
super(id, balance);
this.name = name;
}
public String getName() {
return name;
}
}
```
创建一个有10个账户的数组示例代码如下:
```java
public class ATM {
public static void main(String[] args) {
Newaccount[] accounts = new Newaccount[10];
for (int i = 0; i < accounts.length; i++) {
accounts[i] = new Newaccount(i, 1000, "Account " + i);
}
}
}
```
接下来可以通过调用Account类中的方法来进行存款、取款、查询余额和转账等操作。
相关推荐
















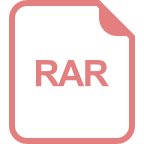
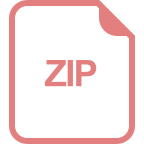
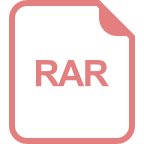