pytest main执行指定用例
时间: 2023-09-13 14:08:29 浏览: 58
执行指定的测试用例可以使用 pytest 的 `-k` 参数,该参数后面跟一个表达式,表示要执行的测试用例名称匹配该表达式的部分。例如,假设你有三个测试用例:test_addition、test_subtraction 和 test_multiplication,你可以通过以下命令只执行 test_addition 和 test_subtraction 两个测试用例:
```
pytest -k "addition or subtraction"
```
在上面的命令中,`-k` 参数后面的表达式为 `"addition or subtraction"`,其中 `or` 表示逻辑或操作。这个表达式会匹配测试用例名称中包含 "addition" 或 "subtraction" 的部分,从而只执行这两个测试用例。
除了使用 `-k` 参数,你也可以通过指定测试文件和测试函数来执行指定的测试用例。例如:
```
pytest test_calculation.py::test_addition
```
这个命令将只执行 `test_calculation.py` 文件中的 `test_addition` 测试函数。
相关问题
golang如何调用pytest执行测试用例
可以使用Go的os/exec包来调用命令行执行pytest测试用例。具体步骤如下:
1. 安装pytest
首先需要在本地安装pytest。可以通过以下命令在命令行中安装:
```
pip install pytest
```
2. 编写Go代码
接下来可以在Go代码中使用os/exec包来执行pytest命令。
```go
package main
import (
"fmt"
"os/exec"
)
func main() {
cmd := exec.Command("pytest")
stdout, err := cmd.Output()
if err != nil {
fmt.Println(err.Error())
return
}
fmt.Println(string(stdout))
}
```
在上面的代码中,我们首先创建了一个名为cmd的命令对象,该对象调用了pytest命令。然后我们使用cmd.Output()方法来执行该命令,并获取它的输出结果。如果执行过程中出现了错误,我们会将错误信息输出到控制台。
3. 执行Go代码
最后,我们可以在命令行中运行我们的Go程序,以调用pytest命令执行测试用例。
```
go run main.go
```
这样就可以使用Go调用pytest执行测试用例了。
pytest标记测试用例并用pytest.main运行
要使用pytest标记测试用例并使用pytest.main运行,你可以在测试用例的函数或类上使用pytest的装饰器或标记,然后在pytest.main的参数中使用这些标记来运行相应的测试用例。
例如,如果你想标记一个测试用例为"smoke",你可以在测试函数上使用@pytest.mark.smoke装饰器。然后,在pytest.main的参数中,使用"-m"选项并指定"smoke"来运行带有该标记的测试用例。
示例代码如下所示:
```pythonimport pytest@pytest.mark.smokedef test_one():
passif __name__ == '__main__':
pytest.main(['-m', 'smoke'])
```
这将只运行带有"smoke"标记的测试用例。你也可以使用其他标记,或者使用多个标记来运行特定的测试用例。
另外,你还可以使用pytest的其他选项来指定要运行的测试用例,例如使用"-k"选项来匹配关键词,或使用"-s"选项来输出详细的测试结果。
希望这能帮助到你! [2 [3
相关推荐
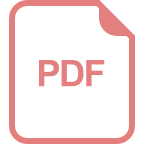
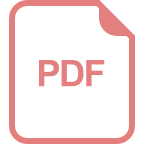












