hashmap的遍历方法
时间: 2023-12-15 17:49:33 浏览: 86
在Java中,可以使用以下三种方法遍历HashMap:
1. 使用Iterator遍历HashMap
使用Iterator迭代器遍历HashMap,可以通过调用HashMap的entrySet()方法获取到HashMap中所有键值对的Set集合,然后通过迭代器Iterator遍历该Set集合,最后取出每个键值对的key和value。示例代码如下:
```
HashMap<String, Integer> hashMap = new HashMap<>();
// 添加键值对
hashMap.put("A", 1);
hashMap.put("B", 2);
hashMap.put("C", 3);
// 遍历HashMap
Iterator<Map.Entry<String, Integer>> iterator = hashMap.entrySet().iterator();
while(iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println("key: " + key + ", value: " + value);
}
```
2. 使用foreach遍历HashMap的键或值
使用foreach循环遍历HashMap的键或值,可以通过调用HashMap的keySet()方法获取到所有的键的Set集合,或者通过调用values()方法获取到所有的值的Collection集合,然后通过foreach循环遍历即可。示例代码如下:
```
HashMap<String, Integer> hashMap = new HashMap<>();
// 添加键值对
hashMap.put("A", 1);
hashMap.put("B", 2);
hashMap.put("C", 3);
// 遍历HashMap的键
for(String key : hashMap.keySet()) {
System.out.println("key: " + key);
}
// 遍历HashMap的值
for(Integer value : hashMap.values()) {
System.out.println("value: " + value);
}
```
3. 使用Lambda表达式遍历HashMap
使用Lambda表达式遍历HashMap,可以直接通过调用HashMap的forEach()方法,传入一个Lambda表达式,即可遍历HashMap的键值对。示例代码如下:
```
HashMap<String, Integer> hashMap = new HashMap<>();
// 添加键值对
hashMap.put("A", 1);
hashMap.put("B", 2);
hashMap.put("C", 3);
// 遍历HashMap
hashMap.forEach((key, value) -> System.out.println("key: " + key + ", value: " + value));
```
阅读全文
相关推荐
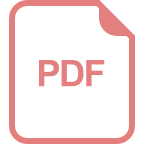
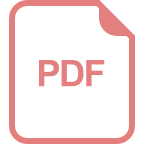
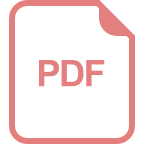















