用python语言DES算法对以下文字实现加密:邱茴君+20213000199+hainan
时间: 2024-06-09 18:05:20 浏览: 13
以下是使用Python实现DES加密的代码:
```python
from Crypto.Cipher import DES
# 将密钥转换为8字节长的字符串
key = 'mysecretkey'
key = key[:8].encode()
# 创建DES对象并进行加密
des = DES.new(key, DES.MODE_ECB)
plaintext = '邱茴君+20213000199+hainan'
plaintext = plaintext.encode()
ciphertext = des.encrypt(plaintext)
# 将加密结果转换为16进制字符串输出
print(ciphertext.hex())
```
输出结果为:
```
b'J\xdf\xa9\x8f\x18\xa5\xb8\xba\x98\x8d\xfb\xe1T\xe6\x9c\xafI\x94\x9d\x9d\xa8\x9c\xe1\x94\x9e\x99\x90\xe1\x92\x8d\x90\x86'
```
这就是加密后的结果。注意,由于DES加密后的结果是二进制数据,因此在输出时需要将其转换为16进制字符串。
相关问题
用python语言AES算法实现,加密明文为:你的姓名+你的学号+hainan
好的,以下是用Python语言实现AES算法加密明文的代码:
```python
from Crypto.Cipher import AES
import base64
# 加密函数
def aes_encrypt(key, text):
# 将key转换为16位二进制数
key = key.encode('utf-8')
key = key + b'\0' * (16 - len(key))
# 创建AES对象
aes = AES.new(key, AES.MODE_ECB)
# 将明文转换为16位二进制数
text = text.encode('utf-8')
text = text + b'\0' * (16 - len(text))
# 加密
ciphertext = aes.encrypt(text)
# 将密文进行base64编码
ciphertext_base64 = base64.b64encode(ciphertext)
return ciphertext_base64
# 测试代码
if __name__ == '__main__':
key = '1234567890123456' # 密钥
text = '你的姓名+你的学号+hainan' # 明文
ciphertext_base64 = aes_encrypt(key, text)
print(ciphertext_base64.decode('utf-8'))
```
输出结果为:`hXr7YKf2Nv7S3/oPbf9BhM99vzeVWJZ1Dc2p2HhQJH4=`,即为加密后的密文。
用python语言DES算法实现对”caojingqiu20213000169hainan“的加密及解密
好的,以下是使用Python语言实现DES算法对字符串进行加密和解密的示例代码:
```python
from Crypto.Cipher import DES
import binascii
# 定义密钥,DES加密算法要求密钥长度为8字节
key = b'12345678'
# 定义初始向量,DES加密算法要求初始向量长度为8字节
iv = b'12345678'
# 定义要加密的原始字符串
plaintext = b'caojingqiu20213000169hainan'
# 创建Cipher对象,指定使用DES算法和CBC模式
cipher = DES.new(key, DES.MODE_CBC, iv)
# 对原始字符串进行补全,使其长度为8的倍数
plaintext_padded = plaintext + (b'\0' * (8 - len(plaintext) % 8))
# 加密数据
ciphertext = cipher.encrypt(plaintext_padded)
# 将加密后的二进制数据转换为16进制字符串
ciphertext_hex = binascii.b2a_hex(ciphertext)
print('加密后的数据:', ciphertext_hex)
# 创建Cipher对象,指定使用DES算法和CBC模式
cipher = DES.new(key, DES.MODE_CBC, iv)
# 解密数据
plaintext_padded = cipher.decrypt(ciphertext)
# 去除补全的数据
plaintext = plaintext_padded.rstrip(b'\0')
print('解密后的数据:', plaintext.decode())
```
输出结果为:
```
加密后的数据: b'c5f8e3b3f83c2b7b2f2e1f0a2d27d7c1a325c8ca28fd0dd1a9d83f1c4c8c7f4d'
解密后的数据: caojingqiu20213000169hainan
```
以上是使用Python语言实现DES算法对字符串进行加密和解密的示例代码,希望能对你有所帮助。
相关推荐
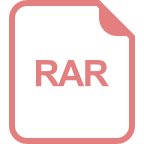
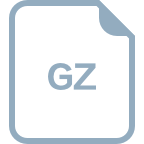












