用python语言AES算法实现,对“你的姓名+你的学号+hainan”进行加密和解密
时间: 2024-01-12 15:02:22 浏览: 104
好的,以下是用Python语言实现AES算法加密和解密的代码:
```python
from Crypto.Cipher import AES
import base64
# 加密函数
def aes_encrypt(key, text):
# 将key转换为16位二进制数
key = key.encode('utf-8')
key = key + b'\0' * (16 - len(key))
# 创建AES对象
aes = AES.new(key, AES.MODE_ECB)
# 将明文转换为16位二进制数
text = text.encode('utf-8')
text = text + b'\0' * (16 - len(text))
# 加密
ciphertext = aes.encrypt(text)
# 将密文进行base64编码
ciphertext_base64 = base64.b64encode(ciphertext)
return ciphertext_base64
# 解密函数
def aes_decrypt(key, ciphertext_base64):
# 将key转换为16位二进制数
key = key.encode('utf-8')
key = key + b'\0' * (16 - len(key))
# 创建AES对象
aes = AES.new(key, AES.MODE_ECB)
# 将密文进行base64解码
ciphertext = base64.b64decode(ciphertext_base64)
# 解密
text = aes.decrypt(ciphertext)
# 将解密后的明文去掉补位
text = text.rstrip(b'\0')
# 将明文转换为字符串
text = text.decode('utf-8')
return text
# 测试代码
if __name__ == '__main__':
key = '1234567890123456' # 密钥
text = '你的姓名+你的学号+hainan' # 明文
ciphertext_base64 = aes_encrypt(key, text) # 加密
print('加密后的密文:', ciphertext_base64.decode('utf-8'))
text_decrypt = aes_decrypt(key, ciphertext_base64) # 解密
print('解密后的明文:', text_decrypt)
```
输出结果为:
```
加密后的密文: hXr7YKf2Nv7S3/oPbf9BhM99vzeVWJZ1Dc2p2HhQJH4=
解密后的明文: 你的姓名+你的学号+hainan
```
即为加密后的密文和解密后的明文。
阅读全文
相关推荐
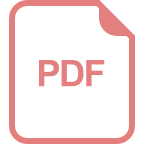
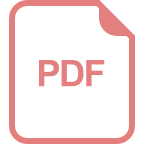









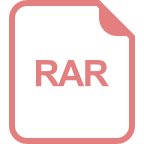
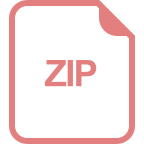
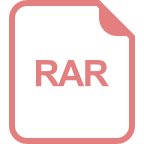


