双摆的python动态图实现
时间: 2024-05-02 14:23:38 浏览: 99
以下是一个简单的双摆模拟的 Python 动态图实现,需要 Matplotlib 库的支持。
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# 定义常量
g = 9.8 # 重力加速度
L1 = 1.0 # 第一根摆杆长度
L2 = 0.5 # 第二根摆杆长度
m1 = 1.0 # 第一根摆杆质量
m2 = 0.5 # 第二根摆杆质量
# 定义初始状态
theta1, theta2 = np.pi / 2, np.pi / 2 # 初始角度
omega1, omega2 = 0.0, 0.0 # 初始角速度
# 定义时间间隔和总时长
dt = 0.05 # 时间间隔
T = 20.0 # 总时长
n = int(T / dt) # 总步数
# 定义动画更新函数
def update(t):
global theta1, theta2, omega1, omega2
# 计算加速度
alpha1 = (-m2 * g * np.sin(theta1 - 2 * theta2) - 2 * m2 * omega2**2 * np.sin(2 * (theta1 - theta2)) - (m1 + m2) * g * np.sin(theta1)) / (L1 * (m1 + m2) - m2 * L1 * np.cos(theta1 - theta2)**2)
alpha2 = (2 * np.sin(theta1 - theta2) * ((m1 + m2) * g * np.cos(theta1) + omega1**2 * L1 * (m1 + m2) + m2 * omega2**2 * L2 * np.cos(theta1 - theta2))) / (L2 * (m1 + m2) - m2 * L2 * np.cos(theta1 - theta2)**2)
# 更新角度和角速度
theta1 += omega1 * dt
theta2 += omega2 * dt
omega1 += alpha1 * dt
omega2 += alpha2 * dt
# 计算摆的坐标
x1 = L1 * np.sin(theta1)
y1 = -L1 * np.cos(theta1)
x2 = x1 + L2 * np.sin(theta2)
y2 = y1 - L2 * np.cos(theta2)
# 清空原有图像
plt.clf()
# 绘制摆的轨迹
plt.plot([0, x1, x2], [0, y1, y2], 'o-', lw=2)
# 设置坐标轴范围和标题
plt.xlim(-1.5, 1.5)
plt.ylim(-1.5, 0.5)
plt.title('Double Pendulum Simulation')
# 初始化图像
fig = plt.figure(figsize=(6, 6))
# 创建动画对象
ani = FuncAnimation(fig, update, frames=np.arange(n), interval=50)
# 显示动画
plt.show()
```
运行上述代码即可看到双摆的动态模拟效果。
阅读全文
相关推荐
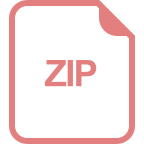
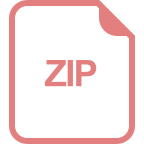
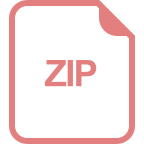
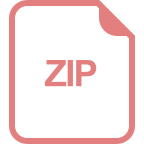
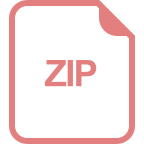
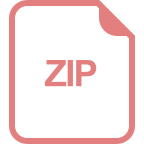
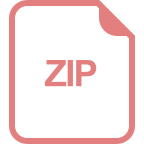
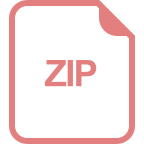
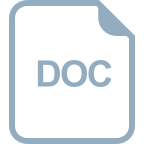
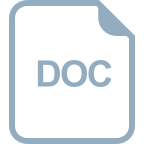
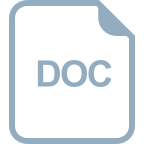
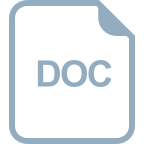
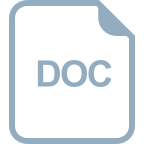
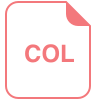


