java上传文件到服务器
时间: 2023-08-09 11:05:31 浏览: 160
可以使用Java中的HttpURLConnection类来实现文件上传到服务器的功能。以下是一个简单的示例代码:
```java
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.file.Files;
public class FileUploader {
public static void main(String[] args) throws IOException {
String serverUrl = "http://example.com/upload";
String filePath = "/path/to/file.txt";
URL url = new URL(serverUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
File file = new File(filePath);
byte[] fileContent = Files.readAllBytes(file.toPath());
try (OutputStream os = conn.getOutputStream()) {
os.write(fileContent);
os.flush();
}
int responseCode = conn.getResponseCode();
System.out.println("Server response code: " + responseCode);
}
}
```
在上面的示例中,我们首先指定了服务器的URL和要上传的文件的路径。然后,我们使用Java中的File类和Files.readAllBytes()方法读取文件的内容,并使用HttpURLConnection类将文件内容发送到服务器。
请注意,在实际应用中,您可能需要添加一些额外的逻辑来处理服务器的响应,例如检查响应代码和内容。
阅读全文
相关推荐
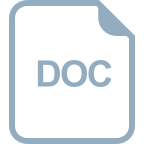
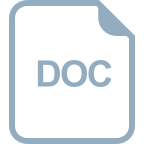
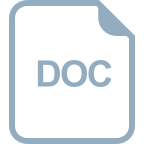
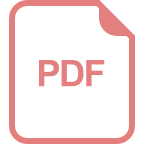
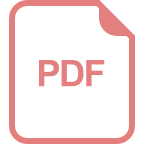
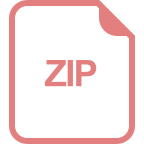
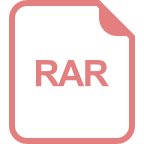
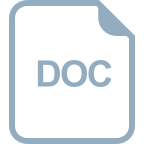
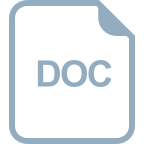
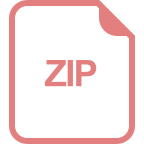
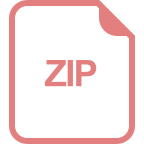
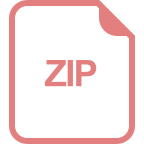
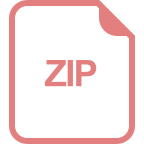
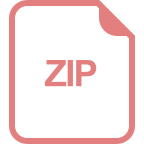
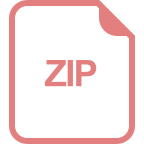
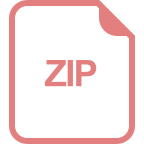