张量内积用pytorch实现
时间: 2023-09-13 20:14:00 浏览: 43
以下是使用PyTorch实现张量内积的示例代码:
```python
import torch
# 创建两个张量
x = torch.tensor([1, 2, 3])
y = torch.tensor([4, 5, 6])
# 计算张量内积
inner_product = torch.dot(x, y)
print("张量 x:", x)
print("张量 y:", y)
print("张量内积:", inner_product)
```
输出结果如下:
```
张量 x: tensor([1, 2, 3])
张量 y: tensor([4, 5, 6])
张量内积: tensor(32)
```
上述代码中,我们使用`torch.dot()`函数计算了张量`x`和`y`的内积,并将结果存储在`inner_product`变量中。最后,我们将张量`x`、张量`y`以及张量内积打印出来。
相关问题
用pytorch 实现张量相加
使用PyTorch实现张量相加也非常简单。您可以使用`torch.add()`函数或直接使用`+`操作符进行张量相加。以下是一个示例:
```python
import torch
tensor1 = torch.tensor([[1, 2, 3], [4, 5, 6]])
tensor2 = torch.tensor([[7, 8, 9], [10, 11, 12]])
# 使用torch.add()函数
result1 = torch.add(tensor1, tensor2)
print(result1)
# 使用+操作符
result2 = tensor1 + tensor2
print(result2)
```
输出:
```
tensor([[ 8, 10, 12],
[14, 16, 18]])
tensor([[ 8, 10, 12],
[14, 16, 18]])
```
希望这可以帮助您使用PyTorch实现张量相加。如果您有任何其他问题,请随时问我。
pytorch实现张量形变
在pytorch中,可以使用view()方法对张量进行形变。view()方法可以根据需要改变张量的形状,但是要保证改变后的形状与原始张量中的元素总数相同。下面是一个示例代码,展示了如何使用view()方法实现张量形变:
```python
import torch
# 创建一个2x3的张量
x = torch.tensor([[1, 2, 3], [4, 5, 6]])
# 将2x3的张量形变为3x2的张量
y = x.view(3, 2)
print(y)
```
输出:
```
tensor([[1, 2],
[3, 4],
[5, 6]])
```
在上面的示例中,我们将一个2x3的张量形变为一个3x2的张量。view()方法根据给定的形状参数,重新排列张量中的元素,得到一个新的形状的张量。
相关推荐














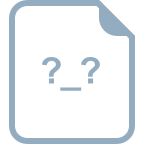
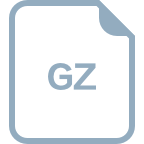