% Define the feedforward function G(s) num = [250, 1250, 4250, 8500]; den = [50, 694, 1690, -739, -3559]; G = tf(num, den); % Define the input function r(t) t = 0:0.01:10; r = ones(size(t)); % Calculate the closed loop transfer function T(s) K = 1 / (1 - 0.41); % Calculate the gain K for a 41% overshoot T = feedback(K * G, 1); % Plot the step response of the closed loop system step(T); % Calculate the closed loop pole locations p = pole(T); disp(p); % Check if a second order approximation is acceptable z = zero(G); wn = sqrt(abs(z(1))^2 + abs(z(2))^2); % Calculate the natural frequency zeta = (-real(z(1))*real(z(2))) / (wn*abs(z(1)+z(2))); % Calculate the damping ratio if zeta >= 0.5 disp("A second order approximation is acceptable."); else disp("A second order approximation is not acceptable."); end
时间: 2024-04-21 21:25:19 浏览: 111
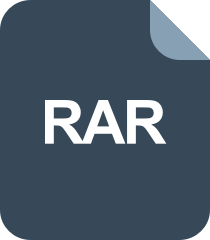
define-CV.rar_The Process
上述代码的输出分为两部分:
1. step(T),即绘制闭环系统的阶跃响应图,这是一个反映系统性能的重要指标。输出为阶跃响应图。
2. disp(p) 和 disp("..."),即输出系统的闭环极点位置和检查是否可以使用二阶近似。输出为闭环系统的极点位置和根据极点位置计算得到的二阶近似是否可行的结果。
阅读全文
相关推荐
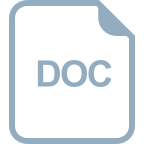
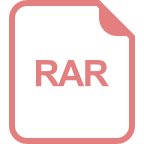
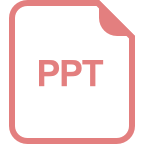
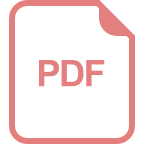
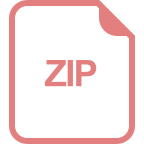
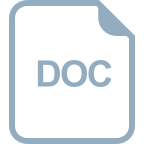
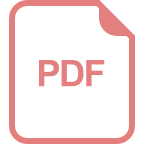
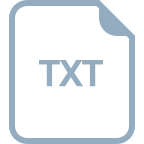
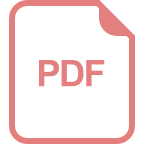
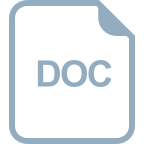
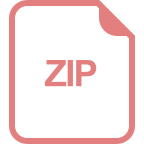
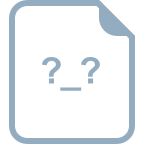
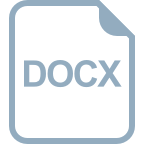
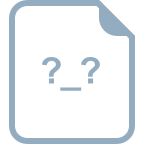
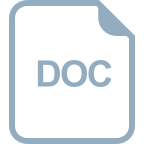

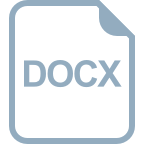