用 for 循环写Define a function even_sum(num), where num is a positive number. The function iterates all the integers numbers from 0 to num, sums them up if they are even numbers, and returns the sum.
时间: 2024-02-04 09:04:14 浏览: 25
好的,下面是用 for 循环实现的 even_sum(num) 函数的代码:
```
def even_sum(num):
sum = 0
for i in range(num+1):
if i % 2 == 0:
sum += i
return sum
```
这个函数的思路是先定义一个 sum 变量,然后用 for 循环遍历 0 到 num 之间的所有整数。在循环中,如果当前的数是偶数,就把它加到 sum 变量中。最后返回 sum 变量的值即可。
相关问题
用 while 循环写Define a function manipulate_list(list1) that returns a new list with 1 added to every element using list comprehension.
好的,以下是使用 while 循环来定义函数 manipulate_list(list1) 的代码:
```python
def manipulate_list(list1):
i = 0
new_list = []
while i < len(list1):
new_list.append(list1[i] + 1)
i += 1
return new_list
```
这个函数将接受一个列表 list1,然后使用 while 循环来遍历列表中的每个元素。在每次循环中,将当前元素加 1,并将其添加到一个新列表 new_list 中。最后,函数将返回这个新列表。
这个函数可以用列表推导式来简化,以下是代码:
```python
def manipulate_list(list1):
new_list = [x + 1 for x in list1]
return new_list
```
这里我们使用列表推导式将一个新列表 new_list 创建为原列表 list1 中每个元素加 1 的结果,并将其作为函数的返回值。
Define a function first_last(list1) that returns a list with the first and last element of list1 interchanged.
Here's the Python code for the function `first_last(list1)` that returns a list with the first and last element of `list1` interchanged:
```python
def first_last(list1):
if len(list1) < 2:
return list1
else:
first = list1[0]
last = list1[-1]
list1[0] = last
list1[-1] = first
return list1
```
This function takes in a list `list1` as its input and first checks if the length of the list is less than 2. If the length is less than 2, it simply returns the original list. Otherwise, it stores the first element of the list in a variable called `first` and the last element of the list in a variable called `last`. It then interchanges the values of `first` and `last` in the list and returns the modified list.
相关推荐
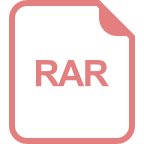
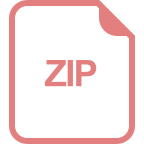












