特征点匹配好的两张图片区域生长算法的代码
时间: 2023-12-22 07:03:37 浏览: 186
以下是一个结合特征点匹配的区域生长算法的代码示例(使用Python语言和OpenCV库实现):
```python
import cv2
import numpy as np
# 定义一个函数用于实现特征点匹配和区域生长
def feature_matching_and_region_growing(img1, img2, seed):
# 创建ORB特征检测器和描述子
orb = cv2.ORB_create()
# 检测特征点和计算描述子
kp1, des1 = orb.detectAndCompute(img1, None)
kp2, des2 = orb.detectAndCompute(img2, None)
# 创建特征点匹配器
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# 进行特征点匹配
matches = bf.match(des1, des2)
# 根据距离进行排序
matches = sorted(matches, key=lambda x: x.distance)
# 获取匹配结果中的坐标
src_pts = np.float32([kp1[m.queryIdx].pt for m in matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in matches]).reshape(-1, 1, 2)
# 使用RANSAC算法进行单应性矩阵估计
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 从种子点开始进行区域生长
rows, cols = img1.shape[:2]
marked = np.zeros_like(img1)
pts = [seed]
threshold = 10
while len(pts) > 0:
cur_pt = pts.pop(0)
cur_pt_homogeneous = np.array([[cur_pt[0], cur_pt[1], 1]], dtype=np.float32)
transformed_pt = cv2.perspectiveTransform(cur_pt_homogeneous, M)[0][0]
transformed_pt = np.round(transformed_pt).astype(int)
if (transformed_pt[0] >= 0 and transformed_pt[0] < cols) and (transformed_pt[1] >= 0 and transformed_pt[1] < rows):
cur_value = img1[cur_pt[1], cur_pt[0]]
transformed_value = img2[transformed_pt[1], transformed_pt[0]]
if abs(cur_value - transformed_value) < threshold and marked[cur_pt[1], cur_pt[0]] == 0:
marked[cur_pt[1], cur_pt[0]] = 255
if cur_pt[0] > 0:
pts.append([cur_pt[0] - 1, cur_pt[1]])
if cur_pt[0] < cols - 1:
pts.append([cur_pt[0] + 1, cur_pt[1]])
if cur_pt[1] > 0:
pts.append([cur_pt[0], cur_pt[1] - 1])
if cur_pt[1] < rows - 1:
pts.append([cur_pt[0], cur_pt[1] + 1])
return marked
# 读入两张图像
img1 = cv2.imread('image1.jpg', 0)
img2 = cv2.imread('image2.jpg', 0)
# 指定一个种子像素点
seed = [50, 50]
# 实现特征点匹配和区域生长
result = feature_matching_and_region_growing(img1, img2, seed)
# 显示结果图像
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
上述代码中,`feature_matching_and_region_growing` 函数首先使用ORB特征检测器和描述子提取图像中的特征点和描述子,然后使用BFMatcher进行特征点匹配。接下来,使用RANSAC算法估计两个图像之间的单应性矩阵,将种子点通过单应性矩阵映射到第二张图像中。最后,在第一张图像上进行区域生长,根据像素点的灰度值差异判断是否需要生长,并将满足条件的像素点加入到生长列表中。最终返回标记图像。
阅读全文
相关推荐
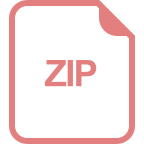
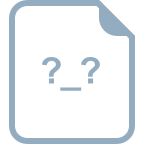
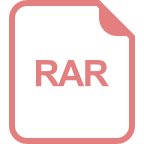
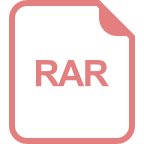
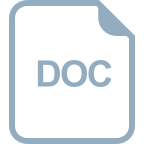
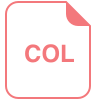
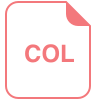
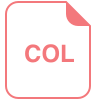
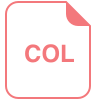
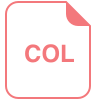
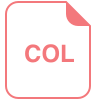
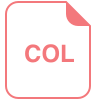
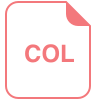
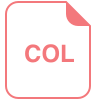
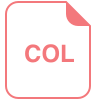
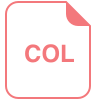
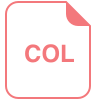
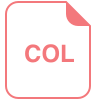
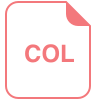