用Java的等待/通知机制实现‘厨师-扯者’扲扴,假擾分沑有6位厨师和6位扯者。厨师做一盘菜的时间捫4s,扯者吃一盘菜的时间捫3s。编程实现这一功能,参考“汾产者-消费者”扲扴。
时间: 2023-05-20 18:01:14 浏览: 91
可以使用Java的wait()和notify()方法来实现这个问题。具体实现步骤如下:
1. 创建一个共享的菜盘对象,用于厨师放菜和扯者取菜。
2. 创建一个厨师线程类,实现Runnable接口,重写run()方法,在run()方法中使用synchronized锁住菜盘对象,判断菜盘是否已经满了,如果已经满了,则调用wait()方法等待扯者取走菜;如果菜盘没有满,则做一盘菜,放到菜盘上,并使用notify()方法通知扯者可以取菜了。
3. 创建一个扯者线程类,实现Runnable接口,重写run()方法,在run()方法中使用synchronized锁住菜盘对象,判断菜盘是否为空,如果为空,则调用wait()方法等待厨师做菜;如果菜盘不为空,则取走一盘菜,吃掉,并使用notify()方法通知厨师可以继续做菜了。
4. 创建6个厨师线程和6个扯者线程,并启动它们。
下面是代码实现:
```
public class ChefWaiterDemo {
public static void main(String[] args) {
Plate plate = new Plate();
for (int i = 0; i < 6; i++) {
new Thread(new Chef(plate)).start();
new Thread(new Waiter(plate)).start();
}
}
}
class Plate {
private int food = 0;
public synchronized void put() throws InterruptedException {
while (food == 6) {
wait();
}
food++;
System.out.println("厨师做了一盘菜,当前菜盘中有" + food + "盘菜");
notifyAll();
}
public synchronized void take() throws InterruptedException {
while (food == 0) {
wait();
}
food--;
System.out.println("扯者吃了一盘菜,当前菜盘中有" + food + "盘菜");
notifyAll();
}
}
class Chef implements Runnable {
private Plate plate;
public Chef(Plate plate) {
this.plate = plate;
}
@Override
public void run() {
try {
while (true) {
Thread.sleep(4000);
plate.put();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
class Waiter implements Runnable {
private Plate plate;
public Waiter(Plate plate) {
this.plate = plate;
}
@Override
public void run() {
try {
while (true) {
Thread.sleep(3000);
plate.take();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
这个程序中,厨师线程每隔4秒做一盘菜,扯者线程每隔3秒吃一盘菜。当菜盘已经满了时,厨师线程调用wait()方法等待扯者线程取走菜;当菜盘为空时,扯者线程调用wait()方法等待厨师线程做菜。使用notifyAll()方法可以通知所有等待的线程,使它们重新竞争锁。
阅读全文
相关推荐
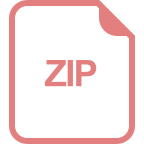
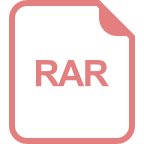
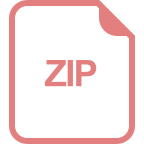
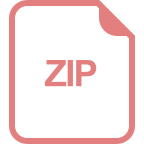
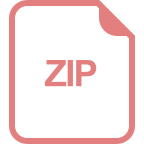
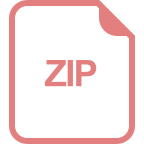
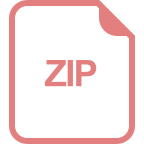
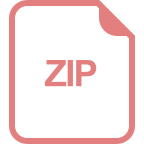
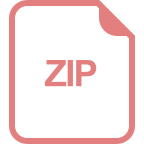
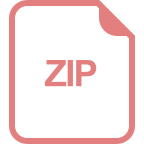
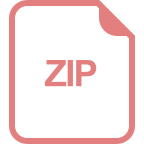
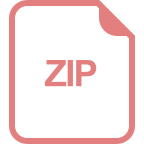
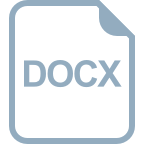
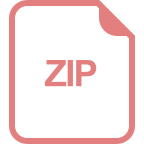
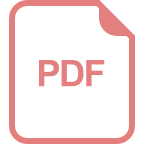
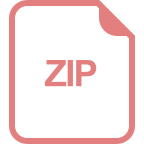
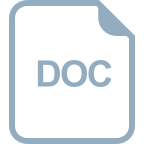