单例模式,工厂模式,观察者模式,MVC模式 ,装饰器模式,适配器模式,命令模式,迭代器模式,策略模式,模板方法模式,这几个设计模式请用java代码写出并做出详细解说
时间: 2023-06-29 19:10:52 浏览: 226
1. 单例模式
单例模式是一种创建型设计模式,用于确保类只有一个实例,并提供全局访问点。
```java
public class Singleton {
private static Singleton instance; //私有化静态对象
private Singleton(){} //私有化构造方法
public static Singleton getInstance(){
if(instance == null){
instance = new Singleton();
}
return instance;
}
}
```
解释:Singleton类只有一个私有的静态实例对象instance,构造方法也是私有的,提供了一个getInstance()方法用于获取该实例,若实例不存在则创建一个新的实例,否则直接返回该实例。
2. 工厂模式
工厂模式是一种创建型设计模式,用于将对象的创建和使用分离开来。
```java
public interface Shape {
void draw();
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Circle.draw()");
}
}
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Square.draw()");
}
}
public class ShapeFactory {
public Shape getShape(String shapeType){
if(shapeType == null){
return null;
}
if(shapeType.equalsIgnoreCase("CIRCLE")){
return new Circle();
} else if(shapeType.equalsIgnoreCase("SQUARE")){
return new Square();
}
return null;
}
}
```
解释:Shape是一个接口,Circle和Square都实现了该接口,并实现了draw()方法。ShapeFactory是一个工厂类,根据传入的参数shapeType来创建对应的对象。
3. 观察者模式
观察者模式是一种行为型设计模式,用于在对象间定义一种一对多的依赖关系,使得一个对象状态的改变能够自动通知并更新其他对象。
```java
public interface Observer {
void update(String message);
}
public interface Subject {
void registerObserver(Observer observer);
void removeObserver(Observer observer);
void notifyObservers();
}
public class MessageBoard implements Subject {
List<Observer> observers = new ArrayList<>();
@Override
public void registerObserver(Observer observer) {
observers.add(observer);
}
@Override
public void removeObserver(Observer observer) {
observers.remove(observer);
}
@Override
public void notifyObservers() {
for (Observer observer : observers) {
observer.update("New message on the board!");
}
}
}
public class User implements Observer {
String name;
public User(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " got the message: " + message);
}
}
```
解释:Observer是观察者接口,定义了update()方法;Subject是主题接口,定义了注册、删除和通知观察者的方法;MessageBoard是一个具体主题类,维护了一个观察者列表,实现了Subject接口;User是观察者类,实现了Observer接口,具有更新方法。
4. MVC模式
MVC模式是一种架构设计模式,用于将应用程序分为三个主要部分:模型、视图和控制器。
```java
public class Model {
private int value;
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
public class View {
public void display(int value){
System.out.println("Value: " + value);
}
}
public class Controller {
private Model model;
private View view;
public Controller(Model model, View view) {
this.model = model;
this.view = view;
}
public void setValue(int value){
model.setValue(value);
view.display(model.getValue());
}
}
```
解释:Model是数据模型,定义了数据的行为;View是用户界面,显示数据和接收用户输入;Controller是控制器,根据用户输入来更新模型并更新视图。
5. 装饰器模式
装饰器模式是一种结构型设计模式,用于动态地将责任附加到对象上。
```java
public interface Shape {
void draw();
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Circle.draw()");
}
}
public abstract class ShapeDecorator implements Shape {
protected Shape decoratedShape;
public ShapeDecorator(Shape decoratedShape) {
this.decoratedShape = decoratedShape;
}
public void draw(){
decoratedShape.draw();
}
}
public class RedShapeDecorator extends ShapeDecorator {
public RedShapeDecorator(Shape decoratedShape) {
super(decoratedShape);
}
public void draw() {
decoratedShape.draw();
setColor(decoratedShape);
}
private void setColor(Shape decoratedShape){
System.out.println("Color: Red");
}
}
```
解释:Shape是一个接口,Circle实现了该接口并实现了draw()方法;ShapeDecorator是一个装饰器抽象类,维护了一个Shape对象,实现了Shape接口并定义了draw()方法;RedShapeDecorator是一个具体装饰器类,扩展了ShapeDecorator,添加了setColor()方法来为Shape对象添加颜色。
6. 适配器模式
适配器模式是一种结构型设计模式,用于将一个类的接口转换为另一个接口,以便于在不同类之间进行交互。
```java
public interface MediaPlayer {
void play(String audioType, String fileName);
}
public interface AdvancedMediaPlayer {
void playVlc(String fileName);
void playMp4(String fileName);
}
public class VlcPlayer implements AdvancedMediaPlayer {
@Override
public void playVlc(String fileName) {
System.out.println("Playing vlc file. Name: " + fileName);
}
@Override
public void playMp4(String fileName) {
// Do nothing
}
}
public class Mp4Player implements AdvancedMediaPlayer{
@Override
public void playVlc(String fileName) {
// Do nothing
}
@Override
public void playMp4(String fileName) {
System.out.println("Playing mp4 file. Name: "+ fileName);
}
}
public class MediaAdapter implements MediaPlayer {
AdvancedMediaPlayer advancedMediaPlayer;
public MediaAdapter(String audioType){
if(audioType.equalsIgnoreCase("vlc") ){
advancedMediaPlayer = new VlcPlayer();
} else if (audioType.equalsIgnoreCase("mp4")){
advancedMediaPlayer = new Mp4Player();
}
}
public void play(String audioType, String fileName) {
if(audioType.equalsIgnoreCase("vlc")){
advancedMediaPlayer.playVlc(fileName);
}else if(audioType.equalsIgnoreCase("mp4")){
advancedMediaPlayer.playMp4(fileName);
}
}
}
public class AudioPlayer implements MediaPlayer {
MediaAdapter mediaAdapter;
public void play(String audioType, String fileName) {
if(audioType.equalsIgnoreCase("mp3")){
System.out.println("Playing mp3 file. Name: " + fileName);
}else if(audioType.equalsIgnoreCase("vlc") || audioType.equalsIgnoreCase("mp4")){
mediaAdapter = new MediaAdapter(audioType);
mediaAdapter.play(audioType, fileName);
}else{
System.out.println("Invalid media. " + audioType + " format not supported");
}
}
}
```
解释:MediaPlayer是一个接口,定义了play()方法;AdvancedMediaPlayer是另一个接口,定义了播放不同格式音频的方法;VlcPlayer和Mp4Player都实现了AdvancedMediaPlayer接口;MediaAdapter是一个适配器类,实现了MediaPlayer接口并包装了AdvancedMediaPlayer对象;AudioPlayer是另一个类,实现了MediaPlayer接口并根据不同的音频格式选择不同的播放方式。
7. 命令模式
命令模式是一种行为型设计模式,用于将请求封装为对象,使得可以用不同的请求来参数化客户端,将请求排队或记录请求日志,以及支持可撤销的操作。
```java
public interface Command {
void execute();
}
public class Light {
public void on(){
System.out.println("Light is on");
}
public void off(){
System.out.println("Light is off");
}
}
public class LightOnCommand implements Command {
Light light;
public LightOnCommand(Light light){
this.light = light;
}
public void execute(){
light.on();
}
}
public class LightOffCommand implements Command {
Light light;
public LightOffCommand(Light light){
this.light = light;
}
public void execute(){
light.off();
}
}
public class RemoteControl {
Command[] onCommands;
Command[] offCommands;
public RemoteControl(){
onCommands = new Command[7];
offCommands = new Command[7];
for (int i = 0; i < 7; i++){
onCommands[i] = () -> {};
offCommands[i] = () -> {};
}
}
public void setCommand(int slot, Command onCommand, Command offCommand){
onCommands[slot] = onCommand;
offCommands[slot] = offCommand;
}
public void onButtonPressed(int slot){
onCommands[slot].execute();
}
public void offButtonPressed(int slot){
offCommands[slot].execute();
}
}
```
解释:Command是一个接口,定义了execute()方法;Light是一个具体类,定义了开灯和关灯的方法;LightOnCommand和LightOffCommand都实现了Command接口,并将Light对象作为构造函数的参数;RemoteControl是一个遥控器类,维护了一个开灯和关灯的命令列表,并提供了绑定命令和执行命令的方法。
阅读全文
相关推荐



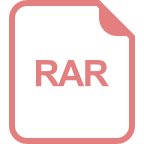

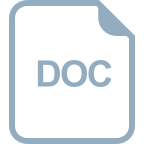











