用C语言写一个贪吃蛇游戏,只能使用标准库函数
时间: 2023-07-20 18:42:03 浏览: 129
好的,下面是一个简单的贪吃蛇游戏代码,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define BOARD_WIDTH 20
#define BOARD_HEIGHT 20
typedef struct _Point {
int x;
int y;
} Point;
typedef struct _Snake {
Point head;
Point tail;
int size;
int direction;
} Snake;
void display(char board[][BOARD_WIDTH + 1], Snake* snake) {
system("cls");
for (int i = 0; i < BOARD_HEIGHT; i++) {
printf("%s\n", board[i]);
}
printf("Score: %d\n", snake->size - 1);
}
void init(char board[][BOARD_WIDTH + 1], Snake* snake) {
for (int i = 0; i < BOARD_HEIGHT; i++) {
for (int j = 0; j < BOARD_WIDTH; j++) {
board[i][j] = ' ';
}
board[i][BOARD_WIDTH] = '\0';
}
snake->head.x = BOARD_WIDTH / 2;
snake->head.y = BOARD_HEIGHT / 2;
snake->tail.x = snake->head.x;
snake->tail.y = snake->head.y;
snake->size = 1;
snake->direction = 0;
board[snake->head.y][snake->head.x] = 'O';
}
Point generate_food(char board[][BOARD_WIDTH + 1]) {
Point food;
do {
food.x = rand() % BOARD_WIDTH;
food.y = rand() % BOARD_HEIGHT;
} while (board[food.y][food.x] != ' ');
board[food.y][food.x] = '@';
return food;
}
int move(Snake* snake, char board[][BOARD_WIDTH + 1]) {
Point new_head;
switch (snake->direction) {
case 0: new_head.x = snake->head.x + 1; new_head.y = snake->head.y; break;
case 1: new_head.x = snake->head.x - 1; new_head.y = snake->head.y; break;
case 2: new_head.x = snake->head.x; new_head.y = snake->head.y - 1; break;
case 3: new_head.x = snake->head.x; new_head.y = snake->head.y + 1; break;
}
if (new_head.x < 0 || new_head.x >= BOARD_WIDTH || new_head.y < 0 || new_head.y >= BOARD_HEIGHT || board[new_head.y][new_head.x] == 'O') {
return 0;
}
if (board[new_head.y][new_head.x] == '@') {
snake->size++;
board[new_head.y][new_head.x] = 'O';
return 1;
}
board[snake->tail.y][snake->tail.x] = ' ';
Point old_tail = snake->tail;
snake->tail = *(Point*)(&board[snake->tail.y][snake->tail.x - 1]);
board[snake->tail.y][snake->tail.x] = 'O';
snake->head = new_head;
*(Point*)(&board[snake->head.y][snake->head.x]) = snake->head;
board[old_tail.y][old_tail.x] = ' ';
return 1;
}
void play() {
char board[BOARD_HEIGHT][BOARD_WIDTH + 1];
Snake snake;
init(board, &snake);
Point food = generate_food(board);
display(board, &snake);
while (1) {
if (_kbhit()) {
char ch = _getch();
if (ch == 'w' && snake.direction != 3) {
snake.direction = 2;
} else if (ch == 's' && snake.direction != 2) {
snake.direction = 3;
} else if (ch == 'a' && snake.direction != 0) {
snake.direction = 1;
} else if (ch == 'd' && snake.direction != 1) {
snake.direction = 0;
}
}
if (!move(&snake, board)) {
printf("Game over!\n");
break;
}
if (snake.size >= BOARD_WIDTH * BOARD_HEIGHT) {
printf("You win!\n");
break;
}
if (snake.size == food.x) {
food = generate_food(board);
}
display(board, &snake);
Sleep(100);
}
}
int main() {
srand((unsigned int)time(NULL));
play();
return 0;
}
```
在Windows平台下,可以使用`conio.h`库中的`_kbhit()`和`_getch()`函数来获取键盘输入。`Sleep()`函数可以用来让程序暂停一段时间,实现动画效果。
该代码中使用了一个字符数组`board`来表示游戏画面,其中空格表示空白区域,`O`表示蛇身,`@`表示食物。`Snake`结构体表示蛇,其中`head`和`tail`表示蛇头和蛇尾的坐标,`size`表示蛇的长度,`direction`表示蛇的移动方向。
`init()`函数用来初始化游戏,将蛇放在画面中央,初始化长度为1,方向向右。`generate_food()`函数用来随机生成食物,并返回其坐标。`move()`函数用来移动蛇,判断蛇是否碰到边界、自身或食物,如果碰到食物,则长度加1,生成新的食物。`play()`函数是游戏主循环,不断监听键盘输入并移动蛇,直到游戏结束。
注意,本代码仅供参考,可能存在不足之处,需要根据实际情况进行修改和完善。
阅读全文
相关推荐
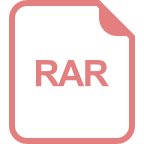

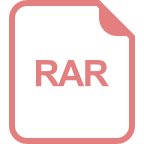













