public List<lsjzsearch> searchId(String id) { /** * @des 按Id查询 * @Author xiaomin * @Date 2023/5/30 * @Param [java.lang.String] * @Return java.util.List<org.example.entity.esEntity.lsjzsearch> */ // 查询 NativeSearchQuery query = new NativeSearchQueryBuilder() .withQuery(QueryBuilders.matchQuery("id", id)) .build(); SearchHits<lsjzsearch> searchHits = elasticsearchTemplate.search(query, lsjzsearch.class); List<lsjzsearch> lsjzsearchList=searchHits.get().map(SearchHit::getContent).collect(Collectors.toList()); for (int i = 0; i < lsjzsearchList.size(); i++) { lsjzsearchList.get(i).setScore(searchHits.getSearchHit(i).getScore()); //设置score属性的值 } return lsjzsearchList; }
时间: 2024-03-29 18:37:16 浏览: 59
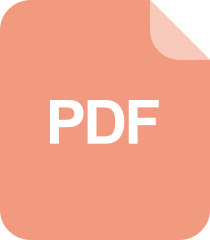
查询数组中的元素-emmc5.1协议
这段代码看起来是在使用 Elasticsearch 进行搜索,根据输入的 id 值查询相关的数据。具体实现是通过构建一个 NativeSearchQuery 对象,设置查询条件为匹配 id 字段的值,然后通过 ElasticsearchTemplate 进行搜索,最后将搜索结果返回成一个 List<lsjzsearch> 类型的列表。其中,对每个搜索结果还设置了一个 score 属性的值。
阅读全文