yolov5添加注意力机制的最好效果的代码展示出来
时间: 2024-04-29 08:21:56 浏览: 26
在 YOLOv5 中添加注意力机制可以提高目标检测的精度和性能。以下是添加注意力机制的代码示例:
```python
# 定义注意力模块
class CBAM(nn.Module):
def __init__(self, planes, reduction_ratio=16, pool_types=['avg', 'max']):
super(CBAM, self).__init__()
self.mlp = nn.Sequential(
OrderedDict([
('fc1', nn.Linear(planes, planes // reduction_ratio)),
('relu1', nn.ReLU()),
('fc2', nn.Linear(planes // reduction_ratio, planes)),
])
)
self.conv_block = nn.Sequential(
nn.Conv2d(in_channels=planes, out_channels=planes, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(planes),
nn.ReLU(),
nn.Conv2d(in_channels=planes, out_channels=planes, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(planes)
)
self.pool_types = pool_types
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.max_pool = nn.AdaptiveMaxPool2d(1)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
module_input = x
batch_size, channels, height, width = x.size()
# Channel Attention Module
avg_pool = self.avg_pool(x)
max_pool = self.max_pool(x)
if 'avg' in self.pool_types:
avg_pool = self.mlp(avg_pool.view(batch_size, -1))
if 'max' in self.pool_types:
max_pool = self.mlp(max_pool.view(batch_size, -1))
channel_att_sum = self.sigmoid(avg_pool + max_pool).unsqueeze(2).unsqueeze(3).expand_as(x)
x = x * channel_att_sum
# Spatial Attention Module
x = module_input * self.sigmoid(self.conv_block(x))
return x
# 定义YOLOv5中的注意力模块
class AttentionBlock(nn.Module):
def __init__(self, in_channels):
super(AttentionBlock, self).__init__()
self.conv_block = nn.Sequential(
nn.Conv2d(in_channels=in_channels, out_channels=in_channels, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(in_channels),
nn.ReLU(),
nn.Conv2d(in_channels=in_channels, out_channels=in_channels, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(in_channels),
nn.ReLU()
)
self.ca = CBAM(in_channels)
self.sa = CBAM(in_channels)
def forward(self, x):
out = self.conv_block(x)
out = self.ca(out) * out
out = self.sa(out) * out
return out
```
然后,在 YOLOv5 中添加注意力机制的代码如下所示:
```python
class YOLOv5(nn.Module):
def __init__(self, num_classes=80, width_mult=1.0, backbone='cspdarknet53'):
super(YOLOv5, self).__init__()
...
self.backbone = nn.Sequential(*backbone_layers)
self.neck = nn.Sequential(
AttentionBlock(in_channels=1024),
SPPBlock(in_channels=1024, out_channels=1024, pool_sizes=[5, 9, 13]),
nn.Conv2d(in_channels=1024, out_channels=512, kernel_size=1),
nn.BatchNorm2d(512),
nn.LeakyReLU(0.1, inplace=True),
nn.Conv2d(in_channels=512, out_channels=1024, kernel_size=3, padding=1),
nn.BatchNorm2d(1024),
nn.LeakyReLU(0.1, inplace=True),
AttentionBlock(in_channels=1024),
nn.Conv2d(in_channels=1024, out_channels=512, kernel_size=1),
nn.BatchNorm2d(512),
nn.LeakyReLU(0.1, inplace=True),
nn.Conv2d(in_channels=512, out_channels=1024, kernel_size=3, padding=1),
nn.BatchNorm2d(1024),
nn.LeakyReLU(0.1, inplace=True),
AttentionBlock(in_channels=1024)
)
self.head = nn.Sequential(
...
)
...
```
在这里,我们添加了三个注意力块,以增强特征图中的重要特征。然后,将注意力模块添加到 YOLOv5 的 neck 部分中。
相关推荐
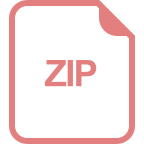
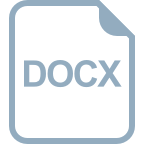
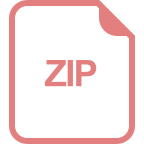














