for i in range(1, dimensions + 1): filename = 'AtrialFibrillationDimension' + str(i) + '_TEST.arff' # change to '_TRAIN.arff' to build training data file = open(filename, "r") dataset = arff.load(file) dataset = np.array(dataset['data']) data.append(dataset[ : , 0 : -1]) data = np.array(data) data = np.transpose(data, (1, 2, 0)) print(data.shape) np.save('X_test.npy', data)
时间: 2024-04-10 07:30:44 浏览: 23
这段代码的目的是从ARFF文件中加载多维时间序列数据,并将其保存为一个numpy数组。
首先,它使用一个循环来遍历维度范围内的数字。在每个迭代中,它构建一个文件名,根据当前维度的索引来选择测试数据集或训练数据集。然后,它打开该文件并使用arff.load函数加载数据集。
接下来,它将加载的数据集转换为numpy数组,并仅保留除最后一列外的所有特征数据。然后,它将这些特征数据添加到data列表中。
在循环结束后,它将data列表转换为numpy数组,并使用np.transpose函数对数据进行转置,以调整维度的顺序。
最后,它打印出data的形状,并使用np.save函数将data保存为名为'X_test.npy'的文件。
这段代码的作用是加载ARFF文件中的多维时间序列数据,并将其保存为numpy数组,以便进行后续的数据处理和分析。
相关问题
pd.read_arff
pandas.read_arff() is a method that reads an ARFF (Attribute-Relation File Format) file and returns a pandas DataFrame.
ARFF files are used in machine learning and data mining tasks to define datasets. They consist of two parts: relation and attributes. The relation part specifies the name of the dataset, while the attribute part defines the attributes of the data, including their names, types, and possible values.
The read_arff() method in pandas allows you to read an ARFF file and convert it into a pandas DataFrame. The resulting DataFrame will have columns corresponding to the attributes defined in the ARFF file, and each row will correspond to an instance of the dataset.
Here's an example usage of the read_arff() method:
```
import pandas as pd
data = pd.read_arff('dataset.arff')
```
This will read the file 'dataset.arff' and return a DataFrame 'data' containing the dataset.
上述代码报错C:\Users\Administrator\AppData\Local\Programs\Python\Python310\lib\site-packages\sklearn\datasets\_openml.py:968: FutureWarning: The default value of `parser` will change from `'liac-arff'` to `'auto'` in 1.4. You can set `parser='auto'` to silence this warning. Therefore, an `ImportError` will be raised from 1.4 if the dataset is dense and pandas is not installed. Note that the pandas parser may return different data types. See the Notes Section in fetch_openml's API doc for details. warn( C:\Users\Administrator\AppData\Local\Programs\Python\Python310\lib\site-packages\sklearn\preprocessing\_encoders.py:868: FutureWarning: `sparse` was renamed to `sparse_output` in version 1.2 and will be removed in 1.4. `sparse_output` is ignored unless you leave `sparse` to its default value. warnings.warn( Traceback (most recent call last): File "D:\pythonProject1\text.py", line 40, in <module> y_sample = y_train[random_index].reshape(1, -1) AttributeError: 'str' object has no attribute 'reshape'
这个错误提示告诉我们,`y_train[random_index]` 是一个字符串类型的对象,而字符串类型没有 `reshape` 方法。因此,我们需要查看代码中对 `y_train` 的处理过程,找到错误的原因并进行修正。
请注意,sklearn 中的许多数据集都是以字符串的形式存储标签的。如果需要对标签进行预处理,我们需要将其转换为数值型数据。通常,我们可以使用 LabelEncoder 或 OneHotEncoder 等方法将标签转换为数值型数据。在这里,您可以尝试添加以下代码对标签进行转换:
```python
from sklearn.preprocessing import LabelEncoder
le = LabelEncoder()
le.fit(y_train)
y_train = le.transform(y_train)
y_sample = le.transform(y_train[random_index]).reshape(1, -1)
```
这里,我们使用 LabelEncoder 将字符串类型的标签转换为数值型数据。注意,如果标签有多个类别,则需要使用 OneHotEncoder 将其转换为二进制形式。
相关推荐
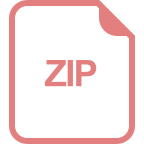












