stm32从一路adc采取的电压值用串口发送出来
时间: 2023-09-09 16:14:03 浏览: 57
好的,您可以先初始化ADC和串口,并设置ADC的采样通道和采样时间。然后在主循环中,使用ADC获取电压值,并发送到串口。以下是示例代码:
```c
#include "stm32f4xx.h"
#include <stdio.h>
#define ADC_PIN GPIO_Pin_0
#define ADC_CHANNEL ADC_Channel_0
void init_adc() {
GPIO_InitTypeDef GPIO_InitStruct;
ADC_InitTypeDef ADC_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
GPIO_InitStruct.GPIO_Pin = ADC_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStruct);
ADC_InitStruct.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStruct.ADC_ScanConvMode = DISABLE;
ADC_InitStruct.ADC_ContinuousConvMode = DISABLE;
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStruct.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStruct);
ADC_Cmd(ADC1, ENABLE);
}
void init_uart() {
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_USART2);
USART_InitStruct.USART_BaudRate = 9600;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Tx;
USART_Init(USART2, &USART_InitStruct);
USART_Cmd(USART2, ENABLE);
}
float read_adc() {
ADC_RegularChannelConfig(ADC1, ADC_CHANNEL, 1, ADC_SampleTime_84Cycles);
ADC_SoftwareStartConv(ADC1);
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
uint16_t adc_value = ADC_GetConversionValue(ADC1);
return adc_value * 3.3f / 4096.0f;
}
void send_uart(char* string) {
for(int i = 0; i < strlen(string); i++) {
USART_SendData(USART2, string[i]);
while(USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET);
}
}
int main() {
init_adc();
init_uart();
while(1) {
float voltage = read_adc();
char buffer[16];
sprintf(buffer, "%.2fV\r\n", voltage);
send_uart(buffer);
}
}
```
在这个例子中,我们将ADC1的通道0连接到PA0引脚。我们初始化了采样时间和分辨率,然后在主循环中使用`read_adc()`函数获取电压值。这个函数将返回一个浮点数,这个浮点数是被读取的电压值。我们将这个值格式化为一个字符串,并使用`send_uart()`函数将它发送到串口中。
希望这可以回答您的问题!
相关推荐
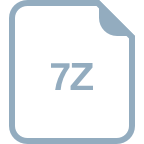
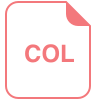
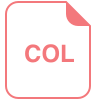
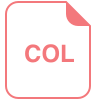
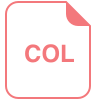
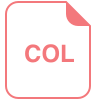









