stm32控制ds18b20
时间: 2023-10-01 11:06:04 浏览: 37
DS18B20是一种数字温度传感器,采用1-wire总线协议进行通信。下面是使用STM32控制DS18B20的基本步骤:
1. 配置GPIO为输出模式,拉低总线至少480us,然后拉高总线至少60us。
2. 等待DS18B20的响应信号,即DS18B20拉低总线15-60us表示准备发送数据。
3. 发送温度转换命令,即向总线发送0x44。
4. 等待转换完成,可以使用延时或者检测DS18B20拉低总线的方式进行等待。
5. 发送读取温度命令,即向总线发送0xBE。
6. 读取DS18B20返回的温度值,温度值为16位的2补码表示,需要进行转换。
以下是一个简单的示例代码:
```c
#include "stm32f4xx.h"
#define DS18B20_GPIO_PORT GPIOB
#define DS18B20_GPIO_PIN GPIO_Pin_12
void DS18B20_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DS18B20_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(500);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(60);
}
void DS18B20_Start(void)
{
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(500);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(60);
}
uint8_t DS18B20_ReadBit(void)
{
uint8_t bit = 0;
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(2);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(12);
bit = GPIO_ReadInputDataBit(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(50);
return bit;
}
uint8_t DS18B20_ReadByte(void)
{
uint8_t i = 0;
uint8_t byte = 0;
for (i = 0; i < 8; i++)
{
byte |= DS18B20_ReadBit() << i;
}
return byte;
}
void DS18B20_WriteByte(uint8_t byte)
{
uint8_t i = 0;
for (i = 0; i < 8; i++)
{
if (byte & (1 << i))
{
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(2);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(60);
}
else
{
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(60);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
Delay_us(2);
}
}
}
float DS18B20_ReadTemperature(void)
{
uint8_t temp_l = 0, temp_h = 0;
int16_t temp = 0;
DS18B20_Start();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0x44);
while (DS18B20_ReadBit() == 0);
DS18B20_Start();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0xBE);
temp_l = DS18B20_ReadByte();
temp_h = DS18B20_ReadByte();
temp = (temp_h << 8) | temp_l;
return (float)temp / 16;
}
```
在使用前需要自行定义延时函数Delay_us()。此代码示例只是提供一个基本的框架,实际使用时需要根据具体需求进行修改。
相关推荐
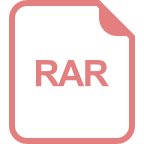














