多头自注意力机制模型的代码
时间: 2024-01-15 12:19:26 浏览: 93
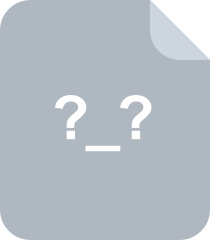
注意力机制代码 python
多头自注意力机制模型的代码可以使用PyTorch来实现。下面是一个示例代码:
```python
import torch
import torch.nn as nn
class MultiHeadAttention(nn.Module):
def __init__(self, d_model, num_heads):
super(MultiHeadAttention, self).__init__()
self.num_heads = num_heads
self.d_model = d_model
self.query_linear = nn.Linear(d_model, d_model)
self.key_linear = nn.Linear(d_model, d_model)
self.value_linear = nn.Linear(d_model, d_model)
self.output_linear = nn.Linear(d_model, d_model)
def forward(self, query, key, value, mask=None):
batch_size = query.size(0)
# 线性变换
query = self.query_linear(query)
key = self.key_linear(key)
value = self.value_linear(value)
# 将输入分割成多个头
query = query.view(batch_size, -1, self.num_heads, self.d_model // self.num_heads).transpose(1, 2)
key = key.view(batch_size, -1, self.num_heads, self.d_model // self.num_heads).transpose(1, 2)
value = value.view(batch_size, -1, self.num_heads, self.d_model // self.num_heads).transpose(1, 2)
# 计算注意力得分
scores = torch.matmul(query, key.transpose(-2, -1)) / torch.sqrt(torch.tensor(self.d_model // self.num_heads, dtype=torch.float32))
if mask is not None:
scores = scores.masked_fill(mask == 0, -1e9)
# 注意力权重
attention_weights = torch.softmax(scores, dim=-1)
# 加权求和
attention_output = torch.matmul(attention_weights, value)
# 将多个头的输出拼接起来
attention_output = attention_output.transpose(1, 2).contiguous().view(batch_size, -1, self.num_heads * (self.d_model // self.num_heads))
# 线性变换
output = self.output_linear(attention_output)
return output
```
这段代码定义了一个名为`MultiHeadAttention`的PyTorch模型类,它包含了多头自注意力机制的实现。在`forward`方法中,首先对输入进行线性变换,然后将输入分割成多个头,计算注意力得分,根据得分计算注意力权重,最后将多个头的输出拼接起来并进行线性变换得到最终的输出。
阅读全文
相关推荐
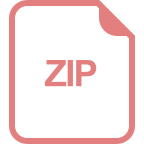

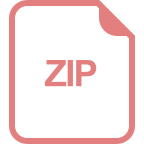
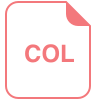
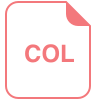











