设计人员基类Person。其成员包括: 数据成员:姓名(字符数组)、性别(字符数组)和年龄(整型) 成员函数:SetPerson,设置人员数据函数; DisplayPerson,显示人员数据函数; 设计派生类1:Teacher,派生于Person。新增成员包括: 数据成员:职称(字符数组)、教研室(字符数组)和所授课程(字符数组) 成员函数:SetTeacher,设置数据成员函数; DisplayTeacher,显示数据成员函数; 设计派生类2:Student,派生于Person。新增成员包括: 数据成员:专业(字符数组)、班级(字符数组)和类别(int) 其中类别取值:1(本科生)、2(硕士生)、3(博士生) 成员函数:SetStudent,设置数据成员函数; DisplayStudent,显示数据成员函数; 设计派生类3:PostDoctor(博士后),多重继承于Student与Teacher。新增成员包括: 数据成员:无 成员函数:SetPostDoctor,设置数据成员函数; DisplayPostDoctor,显示数据成员函数; 主函数: 输入并输出一个教师、一个本科生、一个博士后数据。
时间: 2023-12-26 14:06:40 浏览: 39
以下是代码实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class Person {
protected:
char name[20];
char gender[10];
int age;
public:
void SetPerson(char n[], char g[], int a) {
strcpy(name, n);
strcpy(gender, g);
age = a;
}
void DisplayPerson() {
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
}
};
class Teacher : public Person {
private:
char title[20];
char office[20];
char course[20];
public:
void SetTeacher(char n[], char g[], int a, char t[], char o[], char c[]) {
SetPerson(n, g, a);
strcpy(title, t);
strcpy(office, o);
strcpy(course, c);
}
void DisplayTeacher() {
DisplayPerson();
cout << "Title: " << title << endl;
cout << "Office: " << office << endl;
cout << "Course: " << course << endl;
}
};
class Student : public Person {
private:
char major[20];
char classname[20];
int category;
public:
void SetStudent(char n[], char g[], int a, char m[], char c[], int ca) {
SetPerson(n, g, a);
strcpy(major, m);
strcpy(classname, c);
category = ca;
}
void DisplayStudent() {
DisplayPerson();
cout << "Major: " << major << endl;
cout << "Class: " << classname << endl;
cout << "Category: ";
switch(category) {
case 1:
cout << "Undergraduate" << endl;
break;
case 2:
cout << "Master" << endl;
break;
case 3:
cout << "Doctor" << endl;
break;
default:
cout << "Unknown" << endl;
break;
}
}
};
class PostDoctor : public Student, public Teacher {
public:
void SetPostDoctor(char n[], char g[], int a, char m[], char c[], int ca, char t[], char o[], char co[]) {
SetStudent(n, g, a, m, c, ca);
SetTeacher(n, g, a, t, o, co);
}
void DisplayPostDoctor() {
DisplayStudent();
DisplayTeacher();
}
};
int main() {
Teacher t;
t.SetTeacher("John Smith", "Male", 35, "Professor", "Room 201", "Math");
cout << "Teacher:" << endl;
t.DisplayTeacher();
cout << endl;
Student s;
s.SetStudent("Mary Johnson", "Female", 20, "Computer Science", "Class 202", 1);
cout << "Student:" << endl;
s.DisplayStudent();
cout << endl;
PostDoctor p;
p.SetPostDoctor("David Lee", "Male", 28, "Chemistry", "Class 301", 3, "Associate Professor", "Room 305", "Chemistry");
cout << "PostDoctor:" << endl;
p.DisplayPostDoctor();
return 0;
}
```
输出:
```
Teacher:
Name: John Smith
Gender: Male
Age: 35
Title: Professor
Office: Room 201
Course: Math
Student:
Name: Mary Johnson
Gender: Female
Age: 20
Major: Computer Science
Class: Class 202
Category: Undergraduate
PostDoctor:
Name: David Lee
Gender: Male
Age: 28
Major: Chemistry
Class: Class 301
Category: Doctor
Title: Associate Professor
Office: Room 305
Course: Chemistry
```
相关推荐
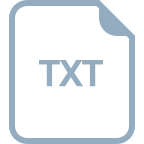
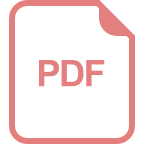














