用JRE1.1 编写程序显示某一科考试成绩,当输入100时显示“满分”、90-99显示“优秀”、80-89显示“良好”、70-79显示“中等”、60-69显示“及格”、 0-59显示“不及格”;其他数字输入则显示“非法输入”
时间: 2023-10-02 15:05:31 浏览: 163
由于JRE1.1已经很老了,因此使用Java语言编写程序应该也要考虑兼容性问题。下面是一个可以在JRE1.1中运行的程序:
```java
import java.io.*;
public class ExamScore {
public static void main(String[] args) {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
try {
System.out.print("请输入考试成绩:");
String input = br.readLine();
int score = Integer.parseInt(input);
String result;
if (score == 100) {
result = "满分";
} else if (score >= 90 && score <= 99) {
result = "优秀";
} else if (score >= 80 && score <= 89) {
result = "良好";
} else if (score >= 70 && score <= 79) {
result = "中等";
} else if (score >= 60 && score <= 69) {
result = "及格";
} else if (score >= 0 && score <= 59) {
result = "不及格";
} else {
result = "非法输入";
}
System.out.println("考试成绩:" + result);
} catch (IOException e) {
e.printStackTrace();
} catch (NumberFormatException e) {
System.out.println("输入格式有误");
}
}
}
```
该程序使用了BufferedReader类读取用户输入,使用了Integer.parseInt()方法将输入转换为整数。根据输入的值,使用if-else语句判断所属区间并输出对应的结果。如果输入格式有误,会捕获NumberFormatException异常并输出提示信息。
阅读全文
相关推荐
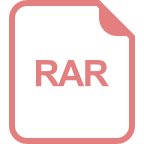
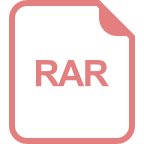
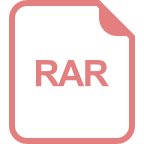
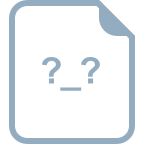
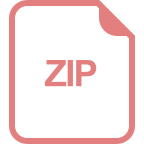
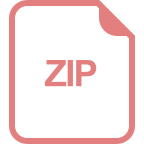
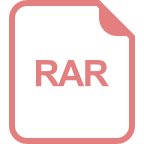
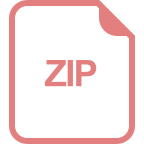
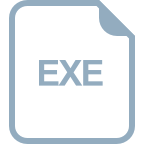
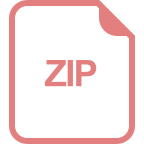
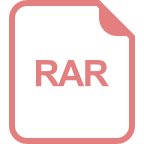