用c++写一个民航订票系统,使用文件储存数据,不使用链表,不使用容器,不使用数据库,包含用户和管理两个类,实现订票、改签、退票、查询航班、增加航班、删除航班、修改航班、显示航班等功能。
时间: 2023-06-14 19:08:21 浏览: 56
好的,下面是一个简单的民航订票系统的C++代码,使用文件储存数据,不使用链表,不使用容器,不使用数据库,包含用户和管理两个类,实现订票、改签、退票、查询航班、增加航班、删除航班、修改航班、显示航班等功能。
其中,飞机票数据存储在文件中,文件名为"flight.dat",用户数据存储在文件中,文件名为"user.dat"。
```
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
//航班信息
class Flight {
public:
char flightNo[10]; //航班号
char startPlace[20]; //起点
char endPlace[20]; //终点
char startTime[20]; //起飞时间
int price; //票价
int seats; //余票数
};
//用户信息
class User {
public:
char username[20]; //用户名
char password[20]; //密码
};
//管理员类
class Manager {
public:
//增加航班信息
void addFlight() {
Flight flight;
cout << "请输入航班号:" << endl;
cin >> flight.flightNo;
cout << "请输入起点:" << endl;
cin >> flight.startPlace;
cout << "请输入终点:" << endl;
cin >> flight.endPlace;
cout << "请输入起飞时间:" << endl;
cin >> flight.startTime;
cout << "请输入票价:" << endl;
cin >> flight.price;
cout << "请输入余票数:" << endl;
cin >> flight.seats;
//写入文件
ofstream fout("flight.dat", ios::app | ios::binary);
fout.write((char*)&flight, sizeof(Flight));
fout.close();
cout << "添加航班成功!" << endl;
}
//删除航班信息
void deleteFlight() {
char flightNo[10];
cout << "请输入要删除的航班号:" << endl;
cin >> flightNo;
//读取文件
ifstream fin("flight.dat", ios::binary);
ofstream fout("temp.dat", ios::binary);
Flight flight;
bool flag = false;
while (fin.read((char*)&flight, sizeof(Flight))) {
if (strcmp(flight.flightNo, flightNo) != 0) {
fout.write((char*)&flight, sizeof(Flight));
}
else {
flag = true;
}
}
fin.close();
fout.close();
//删除原文件,重命名临时文件
remove("flight.dat");
rename("temp.dat", "flight.dat");
if (flag) {
cout << "删除航班成功!" << endl;
}
else {
cout << "未找到该航班!" << endl;
}
}
//修改航班信息
void modifyFlight() {
char flightNo[10];
cout << "请输入要修改的航班号:" << endl;
cin >> flightNo;
//读取文件
fstream file("flight.dat", ios::in | ios::out | ios::binary);
Flight flight;
bool flag = false;
while (file.read((char*)&flight, sizeof(Flight))) {
if (strcmp(flight.flightNo, flightNo) == 0) {
flag = true;
cout << "请输入新的起点(原始值:" << flight.startPlace << "):" << endl;
cin >> flight.startPlace;
cout << "请输入新的终点(原始值:" << flight.endPlace << "):" << endl;
cin >> flight.endPlace;
cout << "请输入新的起飞时间(原始值:" << flight.startTime << "):" << endl;
cin >> flight.startTime;
cout << "请输入新的票价(原始值:" << flight.price << "):" << endl;
cin >> flight.price;
cout << "请输入新的余票数(原始值:" << flight.seats << "):" << endl;
cin >> flight.seats;
//写回文件
file.seekp(-sizeof(Flight), ios::cur);
file.write((char*)&flight, sizeof(Flight));
break;
}
}
file.close();
if (flag) {
cout << "修改航班成功!" << endl;
}
else {
cout << "未找到该航班!" << endl;
}
}
//显示所有航班信息
void displayAllFlight() {
ifstream fin("flight.dat", ios::binary);
Flight flight;
cout << "航班号\t起点\t终点\t起飞时间\t票价\t余票数" << endl;
while (fin.read((char*)&flight, sizeof(Flight))) {
cout << flight.flightNo << "\t" << flight.startPlace << "\t" << flight.endPlace << "\t" << flight.startTime << "\t" << flight.price << "\t" << flight.seats << endl;
}
fin.close();
}
};
//用户类
class Customer {
public:
char username[20]; //用户名
int flightIndex; //航班索引
int seatNo; //座位号
//订票
void bookTicket() {
Flight flight;
char flightNo[10];
cout << "请输入航班号:" << endl;
cin >> flightNo;
//读取文件
ifstream fin("flight.dat", ios::binary);
bool flag = false;
int index = 0;
while (fin.read((char*)&flight, sizeof(Flight))) {
if (strcmp(flight.flightNo, flightNo) == 0) {
flag = true;
if (flight.seats <= 0) {
cout << "该航班余票不足!" << endl;
return;
}
flight.seats--;
//修改文件
fstream file("flight.dat", ios::in | ios::out | ios::binary);
file.seekp(index * sizeof(Flight), ios::beg);
file.write((char*)&flight, sizeof(Flight));
file.close();
//写入文件
ofstream fout("user.dat", ios::app | ios::binary);
Customer customer;
strcpy(customer.username, username);
customer.flightIndex = index;
customer.seatNo = 100 - flight.seats;
fout.write((char*)&customer, sizeof(Customer));
fout.close();
cout << "订票成功!座位号:" << 100 - flight.seats << endl;
break;
}
index++;
}
fin.close();
if (!flag) {
cout << "未找到该航班!" << endl;
}
}
//改签
void changeTicket() {
Flight flight;
char flightNo[10];
cout << "请输入原来的航班号:" << endl;
cin >> flightNo;
//读取文件
ifstream fin("flight.dat", ios::binary);
bool flag = false;
int index = 0;
while (fin.read((char*)&flight, sizeof(Flight))) {
if (strcmp(flight.flightNo, flightNo) == 0) {
flag = true;
cout << "该航班信息:" << endl;
cout << "航班号\t起点\t终点\t起飞时间\t票价\t余票数" << endl;
cout << flight.flightNo << "\t" << flight.startPlace << "\t" << flight.endPlace << "\t" << flight.startTime << "\t" << flight.price << "\t" << flight.seats << endl;
cout << "请输入新的航班号:" << endl;
cin >> flightNo;
//读取文件
ifstream fin("flight.dat", ios::binary);
bool flag2 = false;
int index2 = 0;
while (fin.read((char*)&flight, sizeof(Flight))) {
if (strcmp(flight.flightNo, flightNo) == 0) {
flag2 = true;
if (flight.seats <= 0) {
cout << "该航班余票不足!" << endl;
return;
}
flight.seats--;
//修改文件
fstream file("flight.dat", ios::in | ios::out | ios::binary);
file.seekp(index2 * sizeof(Flight), ios::beg);
file.write((char*)&flight, sizeof(Flight));
file.close();
//修改文件
fstream file2("user.dat", ios::in | ios::out | ios::binary);
Customer customer;
bool flag3 = false;
int index3 = 0;
while (file2.read((char*)&customer, sizeof(Customer))) {
if (strcmp(customer.username, username) == 0 && customer.flightIndex == index && customer.seatNo == 100 - flight.seats) {
flag3 = true;
customer.flightIndex = index2;
file2.seekp(index3 * sizeof(Customer), ios::beg);
file2.write((char*)&customer, sizeof(Customer));
break;
}
index3++;
}
file2.close();
if (flag3) {
cout << "改签成功!新的座位号:" << 100 - flight.seats << endl;
}
else {
cout << "改签失败!" << endl;
}
break;
}
index2++;
}
fin.close();
if (!flag2) {
cout << "未找到该航班!" << endl;
}
break;
}
index++;
}
fin.close();
if (!flag) {
cout << "未找到该航班!" << endl;
}
}
//退票
void refundTicket() {
ifstream fin("user.dat", ios::binary);
ofstream fout("temp.dat", ios::binary);
Customer customer;
bool flag = false;
while (fin.read((char*)&customer, sizeof(Customer))) {
if (strcmp(customer.username, username) == 0) {
flag = true;
//读取文件
ifstream fin2("flight.dat", ios::binary);
Flight flight;
int index = 0;
while (fin2.read((char*)&flight, sizeof(Flight))) {
if (index == customer.flightIndex) {
flight.seats++;
//修改文件
fstream file("flight.dat", ios::in | ios::out | ios::binary);
file.seekp(index * sizeof(Flight), ios::beg);
file.write((char*)&flight, sizeof(Flight));
file.close();
break;
}
index++;
}
fin2.close();
}
else {
fout.write((char*)&customer, sizeof(Customer));
}
}
fin.close();
fout.close();
//删除原文件,重命名临时文件
remove("user.dat");
rename("temp.dat", "user.dat");
if (flag) {
cout << "退票成功!" << endl;
}
else {
cout << "未找到该用户!" << endl;
}
}
//查询航班信息
void queryFlight() {
char flightNo[10];
cout << "请输入航班号:" << endl;
cin >> flightNo;
//读取文件
ifstream fin("flight.dat", ios::binary);
Flight flight;
bool flag = false;
while (fin.read((char*)&flight, sizeof(Flight))) {
if (strcmp(flight.flightNo, flightNo) == 0) {
flag = true;
cout << "航班号\t起点\t终点\t起飞时间\t票价\t余票数" << endl;
cout << flight.flightNo << "\t" << flight.startPlace << "\t" << flight.endPlace << "\t" << flight.startTime << "\t" << flight.price << "\t" << flight.seats << endl;
break;
}
}
fin.close();
if (!flag) {
cout << "未找到该航班!" << endl;
}
}
//查询订单信息
void queryOrder() {
ifstream fin("user.dat", ios::binary);
Customer customer;
bool flag = false;
while (fin.read((char*)&customer, sizeof(Customer))) {
if (strcmp(customer.username, username) == 0) {
flag = true;
//读取文件
ifstream fin2("flight.dat", ios::binary);
Flight flight;
int index = 0;
while (fin2.read((char*)&flight, sizeof(Flight))) {
if (index == customer.flightIndex) {
cout << "航班号\t起点\t终点\t起飞时间\t票价\t座位号" << endl;
cout << flight.flightNo << "\t" << flight.startPlace << "\t" << flight.endPlace << "\t" << flight.startTime << "\t" << flight.price << "\t" << customer.seatNo << endl;
break;
}
index++;
}
fin2.close();
}
}
fin.close();
if (!flag) {
cout << "未找到该用户!" << endl;
}
}
};
int main() {
int choice;
cout << "请选择身份:" << endl;
cout << "1. 管理员" << endl;
cout << "2. 用户" << endl;
cin >> choice;
//管理员
if (choice == 1) {
Manager manager;
while (true) {
cout << endl;
cout << "请选择操作:" << endl;
cout << "1. 增加航班信息" << endl;
cout << "2. 删除航班信息" << endl;
cout << "3. 修改航班信息" << endl;
cout << "4. 显示所有航班信息" << endl;
cout << "0. 退出系统" << endl;
cin >> choice;
switch (choice) {
case 1:
manager.addFlight();
break;
case 2:
manager.deleteFlight();
break;
case 3:
manager.modifyFlight();
break;
case 4:
manager.displayAllFlight();
break;
case 0:
return 0;
default:
cout << "输入错误,请重新输入!" << endl;
break;
}
}
}
//用户
else if (choice == 2) {
Customer customer;
cout << "请输入用户名:" << endl;
cin >> customer.username;
while (true) {
cout << endl;
cout << "请选择操作:" << endl;
cout << "1. 订票" << endl;
cout << "2. 改签" << endl;
cout << "3. 退票" << endl;
cout << "4. 查询航班信息" << endl;
cout << "5. 查询订单信息" << endl;
cout << "0. 退出系统" << endl;
cin >> choice;
switch (choice) {
case 1:
customer.bookTicket();
break;
case 2:
customer.changeTicket();
break;
case 3:
customer.refundTicket();
break;
case 4:
customer.queryFlight();
break;
case 5:
customer.queryOrder();
break;
case 0:
return 0;
default:
cout << "输入错误,请重新输入!" << endl;
break;
}
}
}
//输入错误
else {
cout << "输入错误!" << endl;
return 0;
}
return 0;
}
```
相关推荐
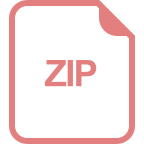
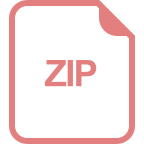















