用c语言写一个接口函数,能够搜索txt文件的内容,并使用链表
时间: 2023-12-10 07:41:53 浏览: 34
以下是一个简单的示例代码,用于搜索txt文件中是否包含特定字符串,并使用链表存储搜索结果:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 1024
// 链表结构体
typedef struct node {
char *line;
struct node *next;
} Node;
// 将搜索结果添加到链表中
void add_result(Node **head, char *line) {
// 创建新节点
Node *new_node = (Node *) malloc(sizeof(Node));
new_node->line = strdup(line);
new_node->next = NULL;
// 如果链表为空,则新节点为头节点
if (*head == NULL) {
*head = new_node;
return;
}
// 否则遍历链表,找到最后一个节点,添加新节点
Node *cur = *head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = new_node;
}
// 搜索txt文件,返回链表
Node *search_txt(char *filename, char *target) {
FILE *fp;
char *line = NULL;
size_t len = 0;
ssize_t read;
Node *head = NULL;
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Unable to open %s\n", filename);
return NULL;
}
// 逐行读取文件,查找是否包含目标字符串
while ((read = getline(&line, &len, fp)) != -1) {
if (strstr(line, target) != NULL) {
add_result(&head, line);
}
}
fclose(fp);
if (line) {
free(line);
}
return head;
}
// 打印链表内容
void print_results(Node *head) {
Node *cur = head;
while (cur != NULL) {
printf("%s", cur->line);
cur = cur->next;
}
}
int main() {
char *filename = "test.txt";
char *target = "apple";
Node *results = search_txt(filename, target);
if (results != NULL) {
printf("Search results:\n");
print_results(results);
} else {
printf("No results found.\n");
}
return 0;
}
```
在此示例代码中,我们首先定义了一个链表结构体 `Node`,它包含一个字符串指针 `line` 和一个指向下一个节点的指针 `next`。接着定义了一个 `add_result` 函数,用于将搜索结果添加到链表中。
在 `search_txt` 函数中,我们打开指定的txt文件,逐行读取该文件并查找是否包含目标字符串。如果发现目标字符串,就调用 `add_result` 函数将该行添加到链表中。最后返回搜索结果链表。
在 `main` 函数中,我们调用 `search_txt` 函数并打印搜索结果。注意,在使用完链表后,需要手动遍历链表并释放每一个节点的内存,防止内存泄漏。
相关推荐
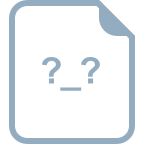
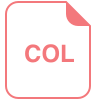
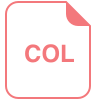
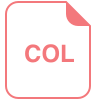
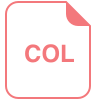










