用C语言创建一个链表,并写出插入和删除的函数
时间: 2023-05-11 09:05:49 浏览: 53
好的,下面是用C语言创建链表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
struct node *head = NULL;
void insert(int data) {
struct node *new_node = (struct node*) malloc(sizeof(struct node));
new_node->data = data;
new_node->next = head;
head = new_node;
}
void delete(int data) {
struct node *current = head;
struct node *previous = NULL;
while (current != NULL && current->data != data) {
previous = current;
current = current->next;
}
if (current == NULL) {
printf("Element not found.\n");
return;
}
if (previous == NULL) {
head = current->next;
} else {
previous->next = current->next;
}
free(current);
}
int main() {
insert(1);
insert(2);
insert(3);
insert(4);
delete(3);
struct node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
return 0;
}
```
这个程序创建了一个链表,并实现了插入和删除函数。插入函数将新节点插入到链表的头部,而删除函数根据给定的数据删除链表中的节点。
相关推荐
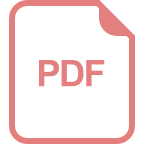
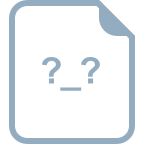
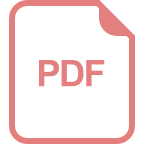














