请用C语言写出哈希表插入和查找函数
时间: 2023-12-24 21:04:16 浏览: 73
好的,以下是哈希表的插入和查找函数的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 哈希表节点结构体
typedef struct hash_node {
char* key; // 键
int value; // 值
struct hash_node* next; // 指向下一个节点的指针
} hash_node;
// 哈希表结构体
typedef struct hash_table {
int size; // 哈希表大小
hash_node** table; // 存储哈希表节点的数组
} hash_table;
// 哈希函数:将字符串转化为整数
unsigned int hash(char* key, int size) {
unsigned int hash_val = 0;
while (*key != '\0') {
hash_val = (hash_val << 5) + *key++;
}
return hash_val % size;
}
// 创建哈希表
hash_table* create_hash_table(int size) {
hash_table* table = (hash_table*) malloc(sizeof(hash_table));
table->size = size;
table->table = (hash_node**) malloc(sizeof(hash_node*) * size);
memset(table->table, 0, sizeof(hash_node*) * size);
return table;
}
// 向哈希表中插入键值对
void insert(hash_table* table, char* key, int value) {
unsigned int index = hash(key, table->size);
hash_node* node = table->table[index];
while (node != NULL && strcmp(node->key, key) != 0) {
node = node->next;
}
if (node == NULL) {
node = (hash_node*) malloc(sizeof(hash_node));
node->key = key;
node->value = value;
node->next = table->table[index];
table->table[index] = node;
} else {
node->value = value;
}
}
// 从哈希表中查找键对应的值
int find(hash_table* table, char* key) {
unsigned int index = hash(key, table->size);
hash_node* node = table->table[index];
while (node != NULL && strcmp(node->key, key) != 0) {
node = node->next;
}
if (node == NULL) {
return -1; // 没有找到
} else {
return node->value;
}
}
// 测试
int main() {
hash_table* table = create_hash_table(100);
insert(table, "apple", 5);
insert(table, "banana", 10);
insert(table, "orange", 15);
printf("apple: %d\n", find(table, "apple"));
printf("banana: %d\n", find(table, "banana"));
printf("orange: %d\n", find(table, "orange"));
printf("grape: %d\n", find(table, "grape"));
return 0;
}
```
上述代码实现了一个简单的哈希表的插入和查找功能,使用了链表处理哈希冲突,可以通过修改哈希函数的实现来适应不同的场景。
阅读全文
相关推荐


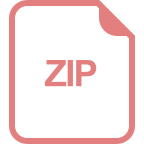
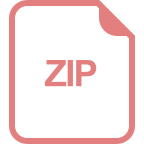
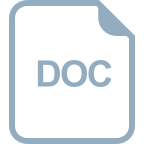









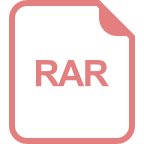
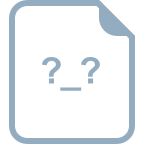