编写链表插入函数Insert将实现链表末尾添加数据,再编写函数Print,实现链表中链表数据打印,编写Destroy函数,实现链表的销毁释放,并在main函数中写出完整的调用过程 C语言
时间: 2023-07-11 17:48:06 浏览: 54
好的,下面是链表插入函数Insert、链表打印函数Print和链表销毁函数Destroy的代码实现,以及main函数的完整调用过程。
```c
#include <stdio.h>
#include <stdlib.h>
// 链表结构体
typedef struct node {
int data; // 数据
struct node* next; // 指向下一个节点的指针
} Node;
// 链表插入函数
void Insert(Node** head, int data) {
// 创建新节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
// 如果链表为空,将新节点作为头节点
if (*head == NULL) {
*head = newNode;
return;
}
// 找到链表的末尾
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
// 将新节点插入到链表末尾
current->next = newNode;
}
// 链表打印函数
void Print(Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
// 链表销毁函数
void Destroy(Node** head) {
Node* current = *head;
while (current != NULL) {
Node* next = current->next;
free(current);
current = next;
}
*head = NULL;
}
int main() {
Node* head = NULL;
// 添加数据到链表末尾
Insert(&head, 1);
Insert(&head, 2);
Insert(&head, 3);
// 打印链表中的数据
printf("链表中的数据为:");
Print(head);
// 销毁链表
Destroy(&head);
return 0;
}
```
在main函数中,先创建一个空链表,然后通过调用Insert函数,在链表末尾添加数据。接着,调用Print函数,将链表中的数据打印出来。最后,调用Destroy函数,销毁链表并释放内存。
相关推荐
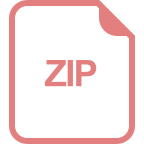
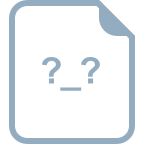
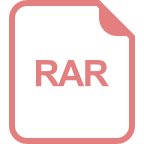












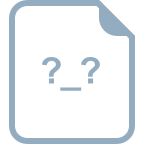
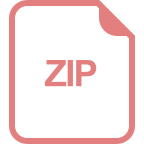
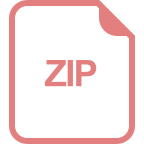