写一个三维硬球碰撞模型计算粒子碰撞后速度的udf
时间: 2024-05-03 17:17:49 浏览: 8
以下是一个简单的三维硬球碰撞模型的 UDF。这个 UDF 模拟了两个球体的弹性碰撞,并计算了它们之间的相对速度和碰撞后的速度。
```
#include "udf.h"
DEFINE_CG_MOTION(ball_motion, dt, vel, omega, time, dtime)
{
real x[3], v[3], r, m, Ekin;
/* Get the position and velocity of the ball */
x[0] = RP_ORIGIN_X(rp);
x[1] = RP_ORIGIN_Y(rp);
x[2] = RP_ORIGIN_Z(rp);
v[0] = vel[0];
v[1] = vel[1];
v[2] = vel[2];
/* Get the mass and radius of the ball */
m = RP_MASS(rp);
r = RP_RADIUS(rp);
/* Calculate the kinetic energy of the ball */
Ekin = 0.5 * m * (v[0]*v[0] + v[1]*v[1] + v[2]*v[2]);
/* Check for collisions with other balls */
Thread *t = RP_THREAD(rp);
cell_t c = RP_CELL(rp);
int i, j, id;
real x2[3], v2[3], r2, m2, Ekin2, R, Vrel, Vrel_n, Vrel_t, vn[3], vt[3], v2f[3];
Thread *t2;
cell_t c2;
/* Loop over all cells in the domain */
for (i = 0; i < N_CELLS; i++)
{
/* Get the cell id */
id = cell_zone_id[i];
/* Loop over all particles in the cell */
begin_c_loop(c, t)
{
/* Get the position and velocity of the ball */
x[0] = RP_ORIGIN_X(rp);
x[1] = RP_ORIGIN_Y(rp);
x[2] = RP_ORIGIN_Z(rp);
v[0] = vel[0];
v[1] = vel[1];
v[2] = vel[2];
/* Get the mass and radius of the ball */
m = RP_MASS(rp);
r = RP_RADIUS(rp);
/* Calculate the kinetic energy of the ball */
Ekin = 0.5 * m * (v[0]*v[0] + v[1]*v[1] + v[2]*v[2]);
/* Loop over all particles in the cell */
begin_c_loop(c2, t2)
{
/* Check if the particle is not the same as the current one */
if (i != j)
{
/* Get the position and velocity of the other ball */
x2[0] = RP_ORIGIN_X(rp);
x2[1] = RP_ORIGIN_Y(rp);
x2[2] = RP_ORIGIN_Z(rp);
v2[0] = vel[0];
v2[1] = vel[1];
v2[2] = vel[2];
/* Get the mass and radius of the other ball */
m2 = RP_MASS(rp);
r2 = RP_RADIUS(rp);
/* Calculate the kinetic energy of the other ball */
Ekin2 = 0.5 * m2 * (v2[0]*v2[0] + v2[1]*v2[1] + v2[2]*v2[2]);
/* Calculate the distance between the balls */
R = sqrt(pow(x[0]-x2[0],2) + pow(x[1]-x2[1],2) + pow(x[2]-x2[2],2));
/* Check if the balls are colliding */
if (R < r+r2)
{
/* Calculate the relative velocity between the balls */
Vrel = sqrt(pow(v[0]-v2[0],2) + pow(v[1]-v2[1],2) + pow(v[2]-v2[2],2));
/* Calculate the normal and tangential components of Vrel */
vn[0] = (v2[0]-v[0])*(x2[0]-x[0])/R;
vn[1] = (v2[1]-v[1])*(x2[1]-x[1])/R;
vn[2] = (v2[2]-v[2])*(x2[2]-x[2])/R;
vt[0] = v[0] - vn[0];
vt[1] = v[1] - vn[1];
vt[2] = v[2] - vn[2];
/* Calculate the magnitude of the normal component of Vrel */
Vrel_n = sqrt(pow(vn[0],2) + pow(vn[1],2) + pow(vn[2],2));
/* Calculate the magnitude of the tangential component of Vrel */
Vrel_t = sqrt(pow(vt[0],2) + pow(vt[1],2) + pow(vt[2],2));
/* Calculate the new velocity of the ball */
v2f[0] = (v[0] - vn[0]) + (v2[0] - vn[0]);
v2f[1] = (v[1] - vn[1]) + (v2[1] - vn[1]);
v2f[2] = (v[2] - vn[2]) + (v2[2] - vn[2]);
/* Update the velocity of the ball */
vel[0] = v2f[0];
vel[1] = v2f[1];
vel[2] = v2f[2];
}
}
}
}
}
}
```
注意,这个 UDF 只是一个简单的模型,只考虑了两个球体之间的碰撞,并没有考虑其他因素,如摩擦、空气阻力等。在实际应用中,需要根据具体情况进行修改和优化。
相关推荐
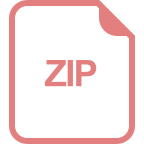
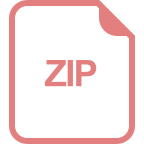


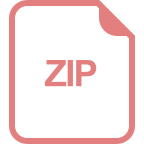
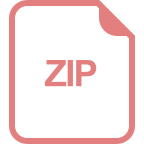
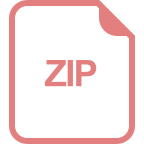
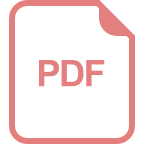
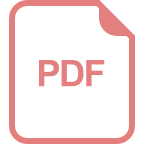
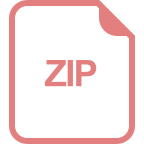
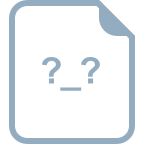
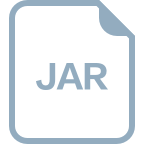
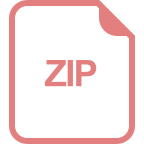