用c++编写一个程序,实现求解带权连通图中最小生成树的算法
时间: 2024-02-05 13:12:05 浏览: 76
好的,下面是使用 Prim 算法求解带权连通图中最小生成树的 C++ 代码:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <cstring>
using namespace std;
const int N = 1e5 + 10, INF = 0x3f3f3f3f;
int head[N], ver[N << 1], edge[N << 1], nxt[N << 1], idx;
bool st[N];
int dist[N], parent[N];
struct Node {
int idx, dist;
bool operator<(const Node &t) const {
return dist > t.dist;
}
};
void add(int a, int b, int c) {
ver[++idx] = b, edge[idx] = c, nxt[idx] = head[a], head[a] = idx;
}
void prim(int n) {
memset(dist, INF, sizeof(dist));
dist[1] = 0;
priority_queue<Node> q;
q.push({1, 0});
while (q.size()) {
auto t = q.top();
q.pop();
int ver = t.idx;
if (st[ver]) continue;
st[ver] = true;
for (int i = head[ver]; i != -1; i = nxt[i]) {
int j = ver[i], k = edge[i];
if (!st[j] && dist[j] > k) {
dist[j] = k;
parent[j] = ver;
q.push({j, dist[j]});
}
}
}
}
int main() {
int n, m;
cin >> n >> m;
memset(head, -1, sizeof(head));
for (int i = 0; i < m; i++) {
int a, b, c;
cin >> a >> b >> c;
add(a, b, c);
add(b, a, c);
}
prim(n);
int sum = 0;
for (int i = 2; i <= n; i++) {
sum += dist[i];
}
cout << sum << endl;
return 0;
}
```
其中,`add()` 函数用于建图,`prim()` 函数实现 Prim 算法,`main()` 函数读入数据,并输出最小生成树的权值和。
在 `prim()` 函数中,我们使用了一个优先队列来维护当前距离集合最近的点。每次取出队首元素,然后遍历其所有相邻的边,将未被访问过的顶点加入队列中。如果该顶点到集合的距离比之前已经记录的更近,则更新该顶点到集合的距离,并记录下其父节点。最后,遍历完所有的顶点,就得到了最小生成树。
阅读全文
相关推荐
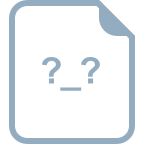
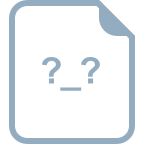



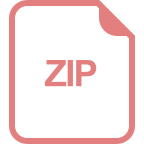

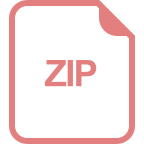
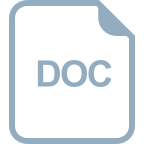
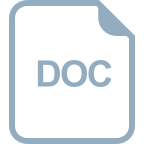
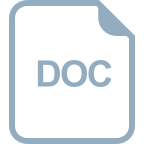
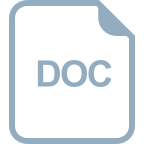



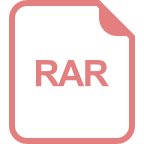