设计一个用于人事管理的类People(人员)。考虑到通用性,这里只抽象出所有类型人员都具有的属性: name(姓名)、sex(性别) 、birthyear(出生年)、birthmonth(出生月)、birthday(出生日) 、id(身份证号)、dept(所在部门)、salary(工资)等等。要求包括下列成员函数:人员信息的录入、人员信息的显示,以及修改和读取各属性信息的公有的成员函数,另外根据需要适当添加构造函数(包括默认的构造函数、带参数的构造函数和拷贝构造函数)和析构函数。实现并测试这个类。
时间: 2023-06-24 15:08:17 浏览: 159
以下是一个可能的People类的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class People {
private:
string name; // 姓名
string sex; // 性别
int birthyear; // 出生年
int birthmonth; // 出生月
int birthday; // 出生日
string id; // 身份证号
string dept; // 所在部门
double salary; // 工资
public:
// 默认构造函数
People() {
name = "";
sex = "";
birthyear = 0;
birthmonth = 0;
birthday = 0;
id = "";
dept = "";
salary = 0.0;
}
// 带参数的构造函数
People(string n, string s, int by, int bm, int bd, string i, string d, double sal) {
name = n;
sex = s;
birthyear = by;
birthmonth = bm;
birthday = bd;
id = i;
dept = d;
salary = sal;
}
// 拷贝构造函数
People(const People& p) {
name = p.name;
sex = p.sex;
birthyear = p.birthyear;
birthmonth = p.birthmonth;
birthday = p.birthday;
id = p.id;
dept = p.dept;
salary = p.salary;
}
// 析构函数
~People() {}
// 人员信息的录入
void input() {
cout << "请输入人员信息:" << endl;
cout << "姓名:";
cin >> name;
cout << "性别:";
cin >> sex;
cout << "出生年月日:";
cin >> birthyear >> birthmonth >> birthday;
cout << "身份证号:";
cin >> id;
cout << "所在部门:";
cin >> dept;
cout << "工资:";
cin >> salary;
}
// 人员信息的显示
void display() const {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "出生日期:" << birthyear << "年" << birthmonth << "月" << birthday << "日" << endl;
cout << "身份证号:" << id << endl;
cout << "所在部门:" << dept << endl;
cout << "工资:" << salary << endl;
}
// 修改姓名
void setName(string n) {
name = n;
}
// 修改性别
void setSex(string s) {
sex = s;
}
// 修改出生年
void setBirthYear(int by) {
birthyear = by;
}
// 修改出生月
void setBirthMonth(int bm) {
birthmonth = bm;
}
// 修改出生日
void setBirthDay(int bd) {
birthday = bd;
}
// 修改身份证号
void setId(string i) {
id = i;
}
// 修改所在部门
void setDept(string d) {
dept = d;
}
// 修改工资
void setSalary(double sal) {
salary = sal;
}
// 读取姓名
string getName() const {
return name;
}
// 读取性别
string getSex() const {
return sex;
}
// 读取出生年
int getBirthYear() const {
return birthyear;
}
// 读取出生月
int getBirthMonth() const {
return birthmonth;
}
// 读取出生日
int getBirthDay() const {
return birthday;
}
// 读取身份证号
string getId() const {
return id;
}
// 读取所在部门
string getDept() const {
return dept;
}
// 读取工资
double getSalary() const {
return salary;
}
};
int main() {
// 创建一个People对象,并输入信息
People p;
p.input();
// 显示信息
p.display();
// 修改姓名和工资
p.setName("张三");
p.setSalary(5000.0);
// 再次显示信息
p.display();
return 0;
}
```
这个类包括了默认构造函数、带参数的构造函数和拷贝构造函数,以及析构函数。它还实现了人员信息的录入、显示和修改各属性信息的公有成员函数。在主函数中,我们创建一个People对象,并测试了其各种功能。
阅读全文
相关推荐


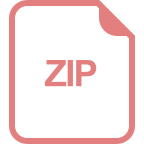









