帮我写一个c语言实现的二叉树查找算法
时间: 2023-06-05 10:06:48 浏览: 107
当然,我很乐意为您提供帮助!以下是一个简单的C语言实现的二叉树查找算法示例:
```
#include<stdio.h>
#include<stdlib.h>
struct node
{
int key;
struct node *left, *right;
};
struct node *newNode(int item)
{
struct node *temp = (struct node *)malloc(sizeof(struct node));
temp->key = item;
temp->left = temp->right = NULL;
return temp;
}
struct node* insert(struct node* node, int key)
{
if (node == NULL) return newNode(key);
if (key < node->key)
node->left = insert(node->left, key);
else if (key > node->key)
node->right = insert(node->right, key);
return node;
}
struct node *search(struct node* root, int key)
{
if (root == NULL || root->key == key)
return root;
if (root->key < key)
return search(root->right, key);
return search(root->left, key);
}
int main()
{
struct node *root = NULL;
root = insert(root, 50);
insert(root, 30);
insert(root, 20);
insert(root, 40);
insert(root, 70);
insert(root, 60);
insert(root, 80);
int x = 60;
struct node* result = search(root, x);
if(result == NULL){
printf("%d does not exist in the tree!", x);
}else{
printf("%d exists in the tree!", result->key);
}
return 0;
}
```
在此示例中,我们创建了一个节点结构体,其中包含一个键值和左右节点指针。 `insert` 函数插入新节点,而 `search` 函数搜索树以找到特定键值。此外,我们还使用 `newNode` 函数来创建新节点,以及使用 `main` 函数为二叉搜索树添加一些节点并搜索特定的值。
祝您编程愉快!
阅读全文
相关推荐
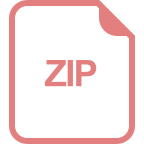
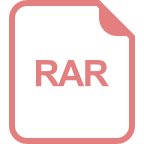
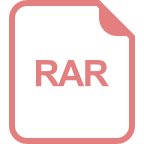
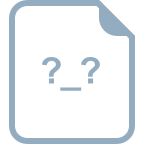
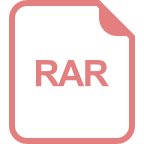
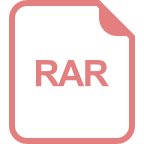
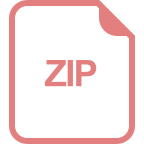
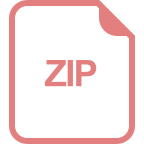
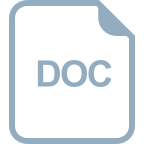
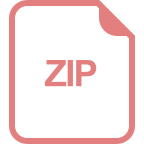
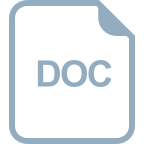
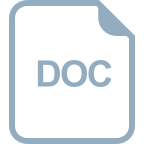
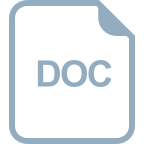
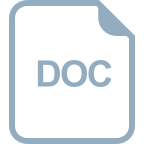
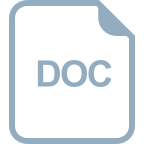
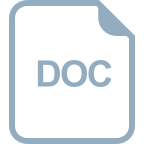