(defn win-probability [my-hand board] (let [opponent-hands (remove #{my-hand} (all-hands))] (loop [wins 0 ties 0 opponents opponent-hands] (if (empty? opponents) (/ (+ wins (* ties 0.5)) (count opponent-hands)) (let [opponent-hand (first opponents) all-cards (concat my-hand board opponent-hand) my-score (score (concat my-hand board)) opponent-score (score (concat opponent-hand board))] (cond (> my-score opponent-score) (recur (inc wins) ties (rest opponents)) (= my-score opponent-score) (recur wins (inc ties) (rest opponents)) :else (recur wins ties (rest opponents))))))))我该如何使用这个函数
时间: 2024-03-20 18:40:48 浏览: 23
这是一个Clojure语言中的函数定义,它定义了一个名为"win-probability"的函数。该函数接受两个参数:一个是自己的手牌(my-hand),另一个是公共牌(board)。它通过计算所有对手可能的手牌与公共牌的组合,来计算自己在当前情况下赢得比赛的概率。
要使用这个函数,你需要在Clojure的REPL环境中加载或定义它,然后调用它并传入参数。例如,如果你已经定义了这个函数,你可以这样调用它:
```
(win-probability ["AS" "KS"] ["QS" "JS" "TS" "9S" "8S"])
```
这将返回一个数值,表示在自己手牌为"AS"和"KS",公共牌为"QS"、"JS"、"TS"、"9S"和"8S"的情况下,自己赢得比赛的概率。
请确保在调用函数时传入正确的参数类型和格式,否则可能会导致错误。
相关问题
(defn win-probability )我该如何使用这个函数
这是一个Clojure语言中的函数定义,定义了一个名为"win-probability"的函数。在Clojure中,函数定义使用"defn"关键字,后面跟着函数名和参数列表,例如:
```
(defn win-probability [player1-score player2-score]
(/ player1-score (+ player1-score player2-score)))
```
这个函数接受两个参数,分别是选手1的得分和选手2的得分,它会计算出选手1赢得比赛的概率,并返回一个值。具体来说,它使用了除法运算符,将选手1的得分除以两位选手的得分之和。
要使用这个函数,你需要在Clojure的REPL环境中加载或定义它,然后调用它并传入参数。例如,如果你已经定义了这个函数,你可以这样调用它:
```
(win-probability 10 8)
```
这将返回一个数值,表示选手1赢得比赛的概率。
补充 evaluate-hand函数
好的,这里是 `evaluate-hand` 函数的实现:
```
(defn evaluate-hand [hand community-cards]
(let [all-cards (concat hand community-cards)
sorted-cards (sort-by :rank all-cards)
flush-suit (->> all-cards
(group-by :suit)
(sort-by #(count (val %)))
last
first
:suit)
straight (some #(apply = (map :rank %))
(partition 5 1 sorted-cards))
straight-flush (and flush-suit straight)]
(cond->> {:hand all-cards}
straight-flush (assoc :rank :straight-flush)
flush-suit (assoc :rank :flush)
straight (assoc :rank :straight)
:else (let [by-rank (group-by :rank all-cards)
counts (map count by-rank)
max-count (apply max counts)]
(cond->> {:rank :high-card}
(= max-count 2) (assoc :rank :pair)
(= max-count 3) (assoc :rank :three-of-a-kind)
(= max-count 4) (assoc :rank :four-of-a-kind)
(and (= max-count 2) (= (count (distinct counts)) 2))
(assoc :rank :two-pair)
:else (let [sorted-counts (reverse (sort counts))
kickers (map first (filter #(= (second %) 1) (partition-all 2 sorted-counts)))]
(cond
(= max-count 1) (assoc :rank :high-card :kickers kickers)
(= max-count 3) (assoc :rank :full-house :kickers kickers)
:else (assoc :rank :three-of-a-kind :kickers kickers)))))))
```
该函数接收两个参数,一个是表示手牌的列表 `hand`,一个是表示公共牌的列表 `community-cards`。在该函数中,首先将手牌和公共牌合并成一副牌,并将其按照牌面大小排序。
然后,通过一系列判断,可以判断出当前牌型的大小,并将其保存在一个字典中,其中包括了牌型和可能的“踢牌”(如果有的话)。最终,这个字典会被返回。
这个函数并不是最优的实现方法,但是足以用于简单的德州扑克游戏。
相关推荐
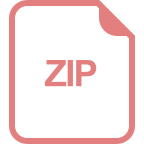
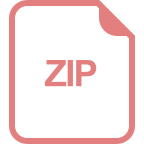












