public static void main(String[] args) { String str = "Hello, world! This is a test sentence."; String englishPunctuations = ",.!?;:\"'()[]<>"; String chinesePunctuations = ",。、!?;:“”‘’()【】《》"; String regex = "[\\p{Punct}&&[^。,、]]"; String replacedStr = str.replaceAll(regex, m -> { String punctuation = m.group(); int index = englishPunctuations.indexOf(punctuation); return index >= 0 ? String.valueOf(chinesePunctuations.charAt(index)) : punctuation; }); System.out.println(replacedStr); // Output: Hello, world! This is a test sentence。 }这段代码提示无法解析group()该如何解决
时间: 2024-01-29 18:02:28 浏览: 308
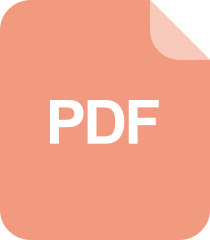
实例分析Java中public static void main(String args[])是什么意思
这个问题可能是因为您没有导入 `java.util.regex` 包中的 `Matcher` 类。您可以在代码开头加上以下导入语句:
```
import java.util.regex.Matcher;
import java.util.regex.Pattern;
```
这样就可以在代码中使用 `Matcher` 类了。完整代码如下:
```
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Main {
public static void main(String[] args) {
String str = "Hello, world! This is a test sentence.";
String englishPunctuations = ",.!?;:\"'()[]<>";
String chinesePunctuations = ",。、!?;:“”‘’()【】《》";
String regex = "[\\p{Punct}&&[^。,、]]";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(str);
String replacedStr = matcher.replaceAll(m -> {
String punctuation = m.group();
int index = englishPunctuations.indexOf(punctuation);
return index >= 0 ? String.valueOf(chinesePunctuations.charAt(index)) : punctuation;
});
System.out.println(replacedStr); // Output: Hello, world! This is a test sentence。
}
}
```
注意,`replaceAll()` 方法需要传入一个 `Matcher` 对象或者一个字符串作为第一个参数。如果传入的是一个字符串,则该方法会直接使用该字符串进行替换,而不需要使用 `group()` 方法。
阅读全文
相关推荐
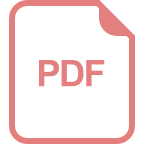

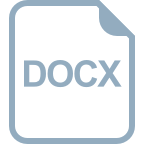
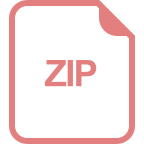
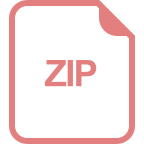
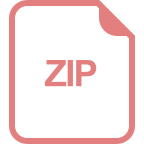
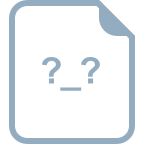
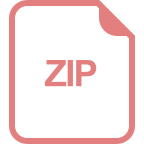
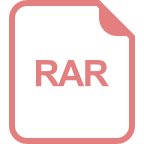
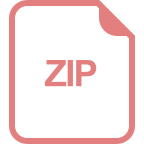