python实现ZZ.random_elementh函数
时间: 2023-07-07 19:34:29 浏览: 221
在数学中,ZZ 表示整数环,random_element 函数可以在给定的整数环中生成一个随机元素。以下是一个 Python 实现 ZZ.random_element 函数的示例代码:
```python
from sage.rings.integer_ring import ZZ
import random
def random_element(n):
"""
生成一个随机元素
:param n: 环的大小
:return: 一个随机整数
"""
return ZZ(random.randint(0, n - 1))
```
该函数使用 SageMath 的 ZZ 类表示整数环,并且使用 Python 自带的 random 模块生成随机整数。函数以整数 n 为参数,返回一个在 [0, n-1] 范围内的随机整数。使用该函数需要安装 SageMath 环境。
相关问题
逻辑回归算法原理python实现
逻辑回归是一种广泛用于分类任务的线性模型,特别适合于二分类问题。其基本思想是建立输入特征和输出概率之间的线性关系,然后使用sigmoid函数将这个线性结果转换为0到1之间的概率,从而预测一个样本属于某个类别的可能性。
Python中,我们可以使用sklearn库中的LogisticRegression模块来实现逻辑回归。以下是逻辑回归的基本步骤:
1. **数据准备**:导入所需的库,如numpy, pandas, 和sklearn,并加载数据集。
```python
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.preprocessing import StandardScaler
# 加载数据(假设数据集名为df)
X = df.drop('target_column', axis=1) # 特征
y = df['target_column'] # 目标变量
```
2. **数据预处理**:通常包括归一化或标准化数据,因为逻辑回归对特征尺度敏感。
```python
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
```
3. **划分训练集和测试集**:
```python
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.2, random_state=42)
```
4. **创建并训练模型**:
```python
model = LogisticRegression(max_iter=10000) # 配置参数,例如迭代次数
model.fit(X_train, y_train)
```
5. **预测和评估**:
```python
y_pred = model.predict(X_test)
accuracy = model.score(X_test, y_test)
```
6. **模型解释**:逻辑回归模型的系数和截距可以用来理解各个特征对目标变量的影响。
用python不使用第三方库实现svm
好的,下面是一个简单的 SVM 实现,包括训练、预测和绘制决策边界。
首先,我们需要导入必要的库:
```python
import numpy as np
import matplotlib.pyplot as plt
```
然后,我们定义一个 SVM 类:
```python
class SVM:
def __init__(self, learning_rate=0.01, regularization_param=0.01, num_iterations=1000):
self.learning_rate = learning_rate
self.regularization_param = regularization_param
self.num_iterations = num_iterations
self.weights = None
self.bias = None
def fit(self, X, y):
num_samples, num_features = X.shape
# Initialize weights and bias
self.weights = np.zeros(num_features)
self.bias = 0
# Gradient descent
for i in range(self.num_iterations):
# Calculate the margin
margin = y * (X.dot(self.weights) + self.bias)
# Calculate the loss
loss = self.regularization_param * np.sum(self.weights ** 2) + np.maximum(0, 1 - margin).mean()
# Calculate the gradients
dW = np.zeros(num_features)
db = 0
if (margin <= 1).all():
dW = self.regularization_param * self.weights - y.dot(X) / num_samples
db = -y.mean()
self.weights -= self.learning_rate * dW
self.bias -= self.learning_rate * db
def predict(self, X):
return np.sign(X.dot(self.weights) + self.bias)
```
其中,`fit` 方法用于训练 SVM 模型,`predict` 方法用于预测。
我们可以使用下面的代码来测试这个 SVM:
```python
# Generate some sample data
np.random.seed(0)
X = np.random.randn(200, 2)
y = np.sign(X[:, 0] ** 2 + X[:, 1] ** 2 - 0.5)
# Train the SVM
svm = SVM()
svm.fit(X, y)
# Plot the decision boundary
xx, yy = np.meshgrid(np.linspace(-3, 3, 500), np.linspace(-3, 3, 500))
zz = svm.predict(np.c_[xx.ravel(), yy.ravel()]).reshape(xx.shape)
plt.contourf(xx, yy, zz, cmap=plt.cm.Paired, alpha=0.8)
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=plt.cm.Paired)
plt.xlim(-3, 3)
plt.ylim(-3, 3)
plt.show()
```
这个 SVM 实现了一个线性核函数。如果需要使用其他核函数,可以将 `X` 转换到一个高维空间,然后使用线性核函数。
阅读全文
相关推荐
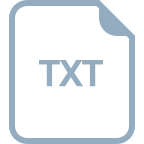
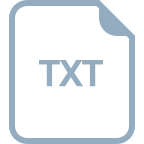
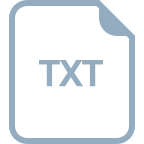








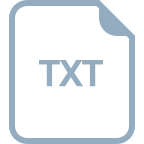
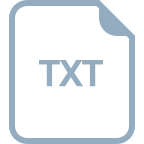
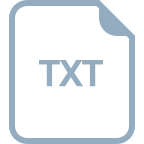
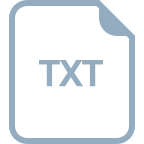
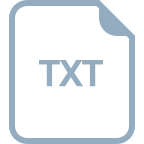
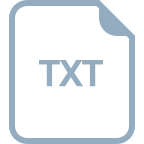