用python生成一段代码,实现注意力机制对一段话进行分类,并标记出话中的关键词
时间: 2024-02-01 08:14:22 浏览: 59
好的,以下是一个简单的示例代码,它使用注意力机制对输入的文本进行分类,并标记出文本中的关键词。
```python
import numpy as np
import tensorflow as tf
# 定义注意力机制函数
def attention(inputs, attention_size):
hidden_size = inputs.shape[2].value # 获取输入的向量维度
W_omega = tf.Variable(tf.random_normal([hidden_size, attention_size], stddev=0.1)) # 定义权重矩阵W
b_omega = tf.Variable(tf.random_normal([attention_size], stddev=0.1)) # 定义偏置向量b
u_omega = tf.Variable(tf.random_normal([attention_size], stddev=0.1)) # 定义参数u
with tf.name_scope('v'):
v = tf.tanh(tf.tensordot(inputs, W_omega, axes=1) + b_omega) # 计算v
# 计算attention权重
vu = tf.tensordot(v, u_omega, axes=1, name='vu')
alphas = tf.nn.softmax(vu, name='alphas')
# 计算加权平均值
output = tf.reduce_sum(inputs * tf.expand_dims(alphas, -1), 1)
return output
# 定义输入数据
input_text = "This is a sample sentence for testing attention mechanism in natural language processing."
input_text = input_text.lower().split()
# 定义词汇表和词向量
vocab = set(input_text)
vocab_size = len(vocab)
word2idx = {w: i for i, w in enumerate(vocab)}
idx2word = {i: w for i, w in enumerate(vocab)}
embedding_dim = 50
embeddings = np.random.randn(vocab_size, embedding_dim)
# 将输入数据转化为词向量
input_indices = [word2idx[w] for w in input_text]
input_vecs = np.array([embeddings[i] for i in input_indices])
# 定义标签和标签向量
labels = ['positive', 'negative']
label2idx = {l: i for i, l in enumerate(labels)}
idx2label = {i: l for i, l in enumerate(labels)}
label_vecs = np.eye(len(labels))
# 定义模型参数
attention_size = 50
hidden_size = 100
output_size = len(labels)
# 定义占位符
inputs = tf.placeholder(tf.float32, [None, None, embedding_dim])
labels = tf.placeholder(tf.float32, [None, output_size])
# 定义模型
with tf.variable_scope('attention'):
attention_output = attention(inputs, attention_size)
with tf.variable_scope('output'):
output = tf.layers.dense(attention_output, hidden_size, activation=tf.nn.relu)
logits = tf.layers.dense(output, output_size)
# 定义损失函数和优化器
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits_v2(labels=labels, logits=logits))
optimizer = tf.train.AdamOptimizer().minimize(loss)
# 进行训练
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(10000):
_, loss_val = sess.run([optimizer, loss], feed_dict={inputs: [input_vecs], labels: [label_vecs[0]]})
if i % 1000 == 0:
print('Step', i, 'loss', loss_val)
# 进行预测
logits_val = sess.run(logits, feed_dict={inputs: [input_vecs]})
pred_idx = np.argmax(logits_val[0])
pred_label = idx2label[pred_idx]
# 标记关键词
attention_val = sess.run(attention_output, feed_dict={inputs: [input_vecs]})
attention_scores = np.squeeze(attention_val, axis=0)
attention_scores = np.abs(attention_scores)
attention_scores /= np.sum(attention_scores)
top_indices = attention_scores.argsort()[-3:][::-1]
top_words = [idx2word[i] for i in top_indices]
print('Predicted label:', pred_label)
print('Top words:', top_words)
```
这段代码使用了注意力机制来提取输入文本中的关键信息,然后将其用于分类。在预测时,代码还标记出了文本中的关键词,以便更好地理解预测结果。
阅读全文
相关推荐
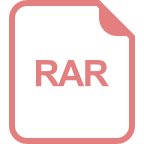
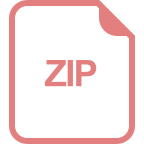
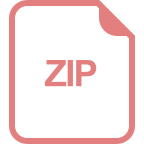
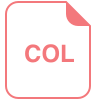
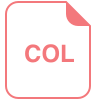
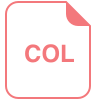
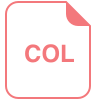
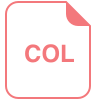
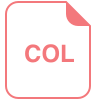
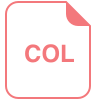
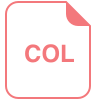
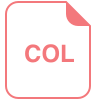
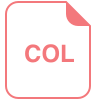
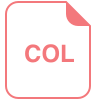
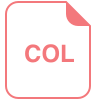
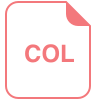
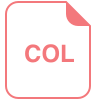