PyMongo插入集合数据代码
时间: 2023-03-31 07:02:35 浏览: 158
可以使用以下代码向集合中插入数据:
```python
from pymongo import MongoClient
# 连接 MongoDB 数据库
client = MongoClient('mongodb://localhost:27017/')
# 选择数据库和集合
db = client['mydatabase']
collection = db['mycollection']
# 插入数据
data = {'name': 'John', 'age': 25}
result = collection.insert_one(data)
# 打印插入数据的 ID
print(result.inserted_id)
```
以上代码会向名为 `mycollection` 的集合中插入一条数据,数据内容为 `{'name': 'John', 'age': 25}`,并打印出插入数据的 ID。
相关问题
pymongo 新建集合
在MongoDB数据库中,使用pymongo库操作数据时,如果你想要新建一个集合(Collection),你可以按照以下步骤进行:
首先,你需要导入`pymongo`模块并连接到你的MongoDB服务器。假设你已经有一个运行中的MongoDB实例,并且你想要连接到名为`your_database`的数据库:
```python
from pymongo import MongoClient
# 创建一个MongoDB客户端
client = MongoClient('mongodb://localhost:27017/')
# 连接到特定的数据库
db = client['your_database']
```
然后,你可以创建一个新的集合,例如`your_collection`,如下所示:
```python
# 新建集合
collection = db.create_collection('your_collection')
```
以上代码会在`your_database`数据库中创建一个新的名为`your_collection`的集合。现在,你可以开始向这个集合插入文档( documents )。
python代码将文档插入mongodb数据库代码
以下是Python代码将文档插入MongoDB数据库的示例:
```python
from pymongo import MongoClient
# 连接到MongoDB服务器
client = MongoClient('mongodb://localhost:27017/')
# 选择数据库
db = client['mydatabase']
# 选择集合
collection = db['mycollection']
# 插入文档
document = {'title': 'MongoDB 教程', 'description': 'MongoDB 是一个 NoSQL 数据库'}
result = collection.insert_one(document)
# 打印插入文档的id
print('插入文档的ID:', result.inserted_id)
```
在上面的示例中,我们使用`pymongo`模块连接到MongoDB服务器,并将`mydatabase`数据库和`mycollection`集合选择为目标。然后我们创建一个名为`document`的字典,其中包含我们要插入的数据。最后,我们使用`insert_one()`方法将文档插入到集合中,并打印插入文档的id。
阅读全文
相关推荐
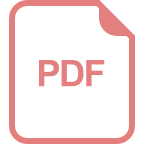
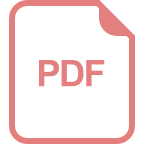
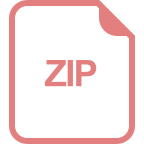
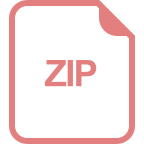
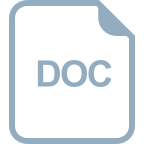
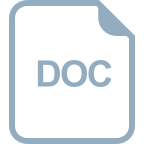
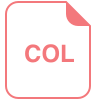
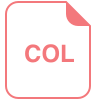
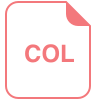
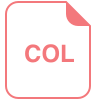
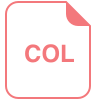
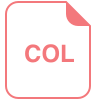
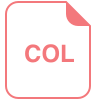


