某学校进行了一次考试,成绩需保存于文件 score.dat。该文件的格式上要求包含两列信息:学号(长整型)、成绩(单精度浮点数)。编与程序,实现信息的录人、保存和显示。 要求: (1)数据录人、保存功能:通过控制台输人若千学生的学号和成绩,將信息保存到文件 score.dat 中。 (2)数据读取、豆示功能:可以从文件 score.dat 中读取学生的学号和成绩,并显示手控制台。
时间: 2024-03-11 07:49:59 浏览: 122
好的,我来为您解答这个问题。
以下是示例代码,您可以根据需要进行修改:
```c++
#include <iostream>
#include <fstream>
using namespace std;
// 定义学生信息结构体
struct Student {
long long id; // 学号
float score; // 成绩
};
// 录入学生信息并保存到文件
void saveToFile() {
ofstream ofs("score.dat", ios::out | ios::binary); // 打开文件
if (!ofs) {
cout << "Failed to open file." << endl;
return;
}
int n;
cout << "Please input the number of students: ";
cin >> n;
Student stu;
for (int i = 0; i < n; i++) {
cout << "Please input the id and score of student " << i+1 << ": ";
cin >> stu.id >> stu.score;
ofs.write((char*)&stu, sizeof(Student)); // 写入文件
}
ofs.close(); // 关闭文件
}
// 从文件中读取学生信息并显示到控制台
void readFromFile() {
ifstream ifs("score.dat", ios::in | ios::binary); // 打开文件
if (!ifs) {
cout << "Failed to open file." << endl;
return;
}
Student stu;
while (ifs.read((char*)&stu, sizeof(Student))) { // 读取文件
cout << "Student id: " << stu.id << ", score: " << stu.score << endl;
}
ifs.close(); // 关闭文件
}
int main() {
saveToFile(); // 录入学生信息并保存到文件
readFromFile(); // 从文件中读取学生信息并显示到控制台
return 0;
}
```
注意事项:
1. 文件名为 score.dat,使用二进制模式打开。
2. 结构体 Student 中的 id 和 score 分别为长整型和单精度浮点数。
3. 写入文件时需要使用指针转换成 char* 类型。
4. 读取文件时需要判断是否读取到了文件末尾。
阅读全文
相关推荐
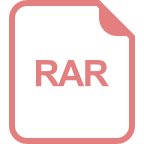
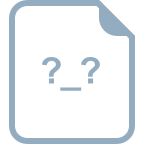







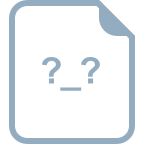
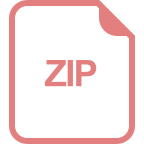
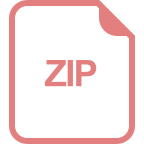
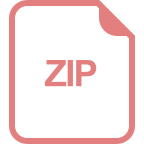
