用编辑软件创建文件score1.txt,存放着某班学生的计算机课成绩(百分制),共有学号、平时成绩、期未成绩三列。请根据平时成绩占40%,期末成绩占60%的比例计算总评成绩,并分学号、总评成绩两列写入另一文件score2.txt。同时在屏幕上输出学生总人数,按总评成绩计90以上、80~89,70~79、 60~69、60分以下各成绩档的人数和班级总平均分(取小数点后两位)。
时间: 2023-06-24 15:07:22 浏览: 99
这是一个比较复杂的任务,需要使用Python来完成。以下是代码实现:
```python
import os
# 读取score1.txt文件
with open('score1.txt', 'r') as f:
lines = f.readlines()
# 计算每个学生的总评成绩,并保存到字典中
students = {}
for line in lines:
student = line.split()
id = student[0]
score = int(student[1]) * 0.4 + int(student[2]) * 0.6
students[id] = score
# 按照总评成绩排序,并写入score2.txt文件
with open('score2.txt', 'w') as f:
for id, score in sorted(students.items(), key=lambda x: x[1], reverse=True):
f.write('{} {}\n'.format(id, score))
# 统计各个成绩档的人数和班级总平均分
n90, n80, n70, n60, nLess60 = 0, 0, 0, 0, 0
total_score = 0
for score in students.values():
if score >= 90:
n90 += 1
elif score >= 80:
n80 += 1
elif score >= 70:
n70 += 1
elif score >= 60:
n60 += 1
else:
nLess60 += 1
total_score += score
n = len(students)
average_score = total_score / n
# 输出统计结果
print('总人数:', n)
print('90分以上:', n90)
print('80~89分:', n80)
print('70~79分:', n70)
print('60~69分:', n60)
print('60分以下:', nLess60)
print('平均分:{:.2f}'.format(average_score))
```
注意,上面代码中的score1.txt文件需要先手动创建,并且每行的格式必须为:学号 平时成绩 期末成绩,以空格分隔。运行代码后,会自动生成score2.txt文件,其中每行的格式为:学号 总评成绩。同时,在屏幕上输出每个成绩档的人数和班级总平均分。
阅读全文
相关推荐
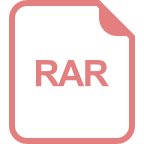










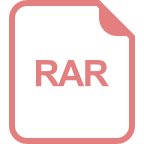
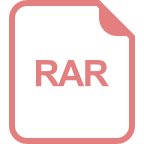
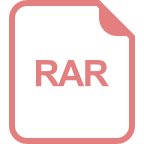
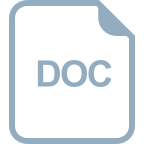