数据为一个excle文件,excle文件有一列随时间变化的LS数据和RS数据,我们使用pytroch里面的lstm模型对LS进行回归预测,用前面50个RS数据和LS数据,预测之后10个LS数据,给出预测评价指标和图片的python代码,不用进行归一化处理
时间: 2024-02-24 15:58:32 浏览: 29
好的,我来给您解答。
首先,我们需要将数据读取进来,并划分为训练集和测试集。以下是读取数据的代码:
```python
import pandas as pd
import numpy as np
data = pd.read_excel('data.xlsx')
LS = data['LS'].values
RS = data['RS'].values
# 划分训练集和测试集
train_LS = LS[:200]
test_LS = LS[200:]
train_RS = RS[:200]
test_RS = RS[200:]
```
接下来,我们需要对数据进行处理,将其转换为模型可以接受的形式。这里我们使用了前50个RS数据和LS数据进行预测,每次预测10个LS数据。以下是处理数据的代码:
```python
def create_dataset(dataset, look_back=50, look_forward=10):
dataX, dataY = [], []
for i in range(len(dataset)-look_back-look_forward+1):
a = dataset[i:(i+look_back)]
dataX.append(np.concatenate((a, train_RS[i:i+look_back]), axis=None))
dataY.append(dataset[i+look_back:i+look_back+look_forward])
return np.array(dataX), np.array(dataY)
trainX, trainY = create_dataset(train_LS)
testX, testY = create_dataset(test_LS)
```
接下来,我们构建LSTM模型进行训练和预测。以下是LSTM模型的代码:
```python
import torch
import torch.nn as nn
class LSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, batch_size, output_dim=1, num_layers=2):
super(LSTM, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.batch_size = batch_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_dim, hidden_dim, num_layers)
self.linear = nn.Linear(hidden_dim, output_dim)
def forward(self, input):
hidden_state = torch.zeros(self.num_layers, self.batch_size, self.hidden_dim)
cell_state = torch.zeros(self.num_layers, self.batch_size, self.hidden_dim)
output, (hidden_state, cell_state) = self.lstm(input.view(len(input), self.batch_size, -1), (hidden_state, cell_state))
predictions = self.linear(output[-1])
return predictions
model = LSTM(input_dim=100, hidden_dim=64, batch_size=1, output_dim=10, num_layers=2)
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
num_epochs = 500
for epoch in range(num_epochs):
train_loss = 0
for i in range(trainX.shape[0]):
optimizer.zero_grad()
X = torch.tensor(trainX[i]).float().view(-1, 1, 100)
Y = torch.tensor(trainY[i]).float().view(-1, 1, 10)
output = model(X)
loss = criterion(output, Y)
loss.backward()
optimizer.step()
train_loss += loss.item()
if epoch % 50 == 0:
print('Epoch {}/{} Train Loss: {:.4f}'.format(epoch+1, num_epochs, train_loss/trainX.shape[0]))
```
最后,我们对模型进行预测,并计算评价指标。以下是预测和评价指标的代码:
```python
from sklearn.metrics import mean_squared_error, r2_score
import matplotlib.pyplot as plt
# 预测
predictions = []
with torch.no_grad():
for i in range(testX.shape[0]):
X = torch.tensor(testX[i]).float().view(-1, 1, 100)
output = model(X)
predictions.append(output.view(-1))
predictions = np.array(predictions).reshape(-1, 10)
# 评价指标
mse = mean_squared_error(testY, predictions)
r2 = r2_score(testY, predictions)
print('MSE: {:.4f}'.format(mse))
print('R2 score: {:.4f}'.format(r2))
# 绘制预测图像
plt.figure(figsize=(10, 6))
plt.plot(testY.reshape(-1), label='True')
plt.plot(predictions.reshape(-1), label='Predicted')
plt.legend()
plt.show()
```
这样就完成了问题的解答。
相关推荐
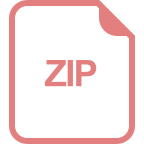











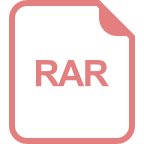
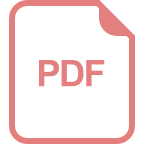
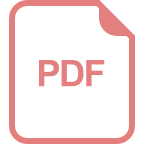