写一个C++代码,要求如下:将学生信息写入文本文件。一个学生的信息包括姓名和成绩,这些信息分别写入文件。要求:(1)学生的信息用一个Student类的对象表示,包含string firstName、c...
时间: 2024-02-12 21:09:18 浏览: 72
好的,以下是符合要求的C++代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Student {
private:
string firstName;
char lastNameInitial;
int score;
public:
Student(string first, char last, int s) {
firstName = first;
lastNameInitial = last;
score = s;
}
void print() {
cout << firstName << " " << lastNameInitial << ".: " << score << endl;
}
string getFirstName() {
return firstName;
}
char getLastNameInitial() {
return lastNameInitial;
}
int getScore() {
return score;
}
};
int main() {
// 创建Student对象
Student s1("Tom", 'S', 85);
Student s2("Jerry", 'M', 92);
Student s3("Lucy", 'B', 76);
// 将学生信息写入文件
ofstream outfile("students.txt");
outfile << s1.getFirstName() << " " << s1.getLastNameInitial() << ".: " << s1.getScore() << endl;
outfile << s2.getFirstName() << " " << s2.getLastNameInitial() << ".: " << s2.getScore() << endl;
outfile << s3.getFirstName() << " " << s3.getLastNameInitial() << ".: " << s3.getScore() << endl;
outfile.close();
// 从文件中读取学生信息
ifstream infile("students.txt");
string line;
while (getline(infile, line)) {
cout << line << endl;
}
infile.close();
return 0;
}
```
这段代码定义了一个Student类,用于表示学生信息。每个Student对象有三个属性:firstName(名字)、lastNameInitial(姓氏首字母)和score(成绩)。在main函数中,创建了三个Student对象并将它们的信息写入文件。然后从文件中读取学生信息并输出到控制台。
阅读全文
相关推荐
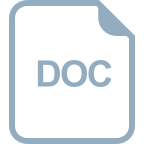
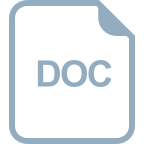
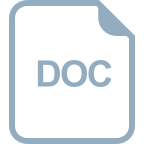
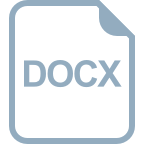
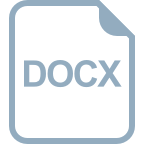
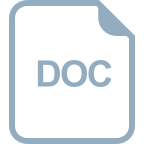
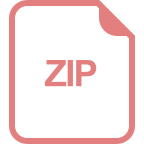
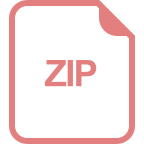
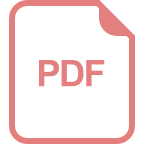
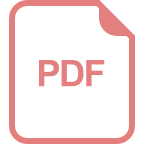
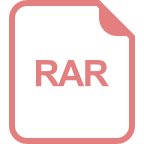
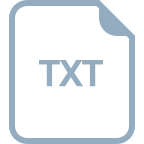
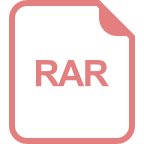
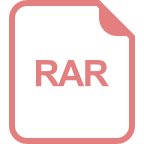
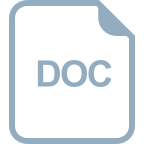
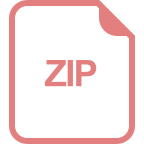