如何手机和电脑通讯字符串(用C#语言)
时间: 2024-05-05 15:16:18 浏览: 105
语言举例)?
可以使用串口通讯的方式,通过在手机和电脑之间建立一个串口连接,将数据以字符串的形式传输。在C语言中,可以使用串口通讯库如“serial.h”来实现串口通讯。下面是一个简单的例子:
1. 在电脑上打开一个串口连接,指定波特率和其他相关参数,如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
int open_port(void)
{
int fd;
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
perror("open_port: Unable to open /dev/ttyUSB0");
}
else
{
fcntl(fd, F_SETFL, 0);
}
return (fd);
}
int set_port_attribs(int fd, int speed, int parity)
{
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(fd, &tty) != 0)
{
perror("error %d from tcgetattr");
return -1;
}
cfsetospeed(&tty, speed);
cfsetispeed(&tty, speed);
tty.c_cflag = (tty.c_cflag & ~CSIZE) | CS8; // 8-bit characters
// disable IGNBRK for mismatched speed tests; otherwise receive break
// as \000 chars
tty.c_iflag &= ~IGNBRK; // disable break processing
tty.c_lflag = 0; // no signaling chars, no echo, no canonical processing
tty.c_oflag = 0; // no remapping, no delays
tty.c_cc[VMIN] = 0; // read doesn't block
tty.c_cc[VTIME] = 5; // 0.5 seconds read timeout
tty.c_iflag &= ~(IXON | IXOFF | IXANY); // shut off xon/xoff ctrl
tty.c_cflag |= (CLOCAL | CREAD); // ignore modem controls, enable reading
tty.c_cflag &= ~(PARENB | PARODD); // shut off parity
tty.c_cflag |= parity;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CRTSCTS;
if (tcsetattr(fd, TCSANOW, &tty) != 0)
{
perror("error %d from tcsetattr");
return -1;
}
return 0;
}
void set_blocking(int fd, int should_block)
{
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(fd, &tty) != 0)
{
perror("error %d from tggetattr");
return;
}
tty.c_cc[VMIN] = should_block ? 1 : 0;
tty.c_cc[VTIME] = 5; // 0.5 seconds read timeout
if (tcsetattr(fd, TCSANOW, &tty) != 0)
perror("error %d setting term attributes");
}
int main()
{
int fd = open_port();
if (fd < 0)
{
exit(1);
}
set_port_attribs(fd, B9600, 0); // set speed to 9600 bps, 8n1 (no parity)
set_blocking(fd, 0); // set no blocking
char buf[256];
int n;
while (1)
{
n = read(fd, buf, sizeof(buf));
if (n > 0)
{
buf[n] = 0;
printf("%s", buf);
}
}
}
```
2. 在手机端,可以使用Android的串口通讯库,如“android-serialport-api”,来实现串口通讯。
下面是一个简单的例子:
```c
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <jni.h>
#include "android/log.h"
#include "SerialPort.h"
#define LOG_TAG "SerialPort"
#define LOGD(...) __android_log_print(ANDROID_LOG_DEBUG, LOG_TAG, __VA_ARGS__)
#define LOGE(...) __android_log_print(ANDROID_LOG_ERROR, LOG_TAG, __VA_ARGS__)
int main()
{
SerialPort* serialPort = new SerialPort("/dev/ttyUSB1", 9600, 0);
if (serialPort == NULL)
{
LOGE("Failed to open serial port");
exit(1);
}
char buf[256];
int n;
while (1)
{
n = serialPort->Read(buf, sizeof(buf));
if (n > 0)
{
buf[n] = 0;
LOGD("%s", buf);
}
}
}
```
阅读全文
相关推荐



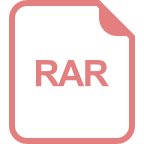






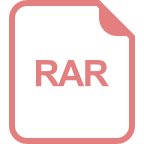

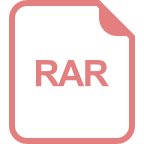

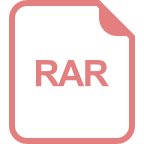


