java sm4
时间: 2023-10-04 09:12:15 浏览: 79
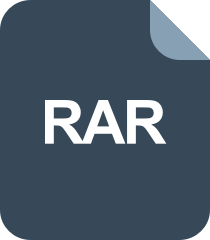
sm2、SM3、sm4国密加密算法java实现.rar
Java SM4是一种对称加密算法,它使用固定长度(128位)的密钥对数据进行加密和解密。Java SM4算法的实现可以使用Bouncy Castle等Java密码库来进行。
以下是使用Bouncy Castle实现Java SM4加密和解密的示例代码:
```java
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.modes.CBCBlockCipher;
import org.bouncycastle.crypto.paddings.PKCS7Padding;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import org.bouncycastle.util.encoders.Hex;
import java.nio.charset.StandardCharsets;
import java.security.Security;
public class SM4Example {
public static void main(String[] args) throws Exception {
// 加载Bouncy Castle库
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
// 待加密的明文
String plaintext = "Hello world!";
byte[] plainBytes = plaintext.getBytes(StandardCharsets.UTF_8);
// 128位密钥
String keyHex = "0123456789abcdeffedcba9876543210";
byte[] keyBytes = Hex.decode(keyHex);
// 128位初始向量
String ivHex = "0123456789abcdef0123456789abcdef";
byte[] ivBytes = Hex.decode(ivHex);
// 加密
CBCBlockCipher cipher = new CBCBlockCipher(new SM4Engine());
PKCS7Padding padding = new PKCS7Padding();
cipher.init(true, new ParametersWithIV(new KeyParameter(keyBytes), ivBytes));
byte[] ciphertext = new byte[cipher.getOutputSize(plainBytes.length)];
int len = cipher.processBytes(plainBytes, 0, plainBytes.length, ciphertext, 0);
len += cipher.doFinal(ciphertext, len);
System.out.println("Ciphertext: " + Hex.toHexString(ciphertext));
// 解密
cipher.init(false, new ParametersWithIV(new KeyParameter(keyBytes), ivBytes));
byte[] decrypted = new byte[cipher.getOutputSize(len)];
int decryptedLen = cipher.processBytes(ciphertext, 0, len, decrypted, 0);
decryptedLen += cipher.doFinal(decrypted, decryptedLen);
String decryptedText = new String(decrypted, 0, decryptedLen, StandardCharsets.UTF_8);
System.out.println("Decrypted text: " + decryptedText);
}
}
```
在这个示例中,我们使用Bouncy Castle库来实现Java SM4算法的加密和解密,并使用16字节(128位)的密钥和初始向量。加密过程使用CBC模式和PKCS7填充方式。
阅读全文
相关推荐
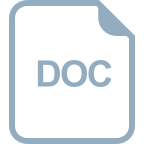
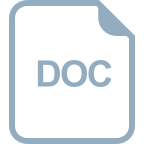








