使用angular开发时,用nz zorro里面的table组建,发现分页器变成了竖版,怎么解决
时间: 2024-02-21 13:00:54 浏览: 236
如果您使用的是 ng-zorro-antd 的早期版本,您可以将 `<nz-table>` 组件的 `[nzPageSizeOptions]` 属性设置为空数组来禁用竖版分页器,例如:
```html
<nz-table [nzData]="data" [nzPageSize]="10" [nzPageSizeOptions]="[]">
<!-- 这里是表格内容 -->
</nz-table>
```
如果您使用的是 ng-zorro-antd 的更新版本,您可以使用 `[nzShowSizeChanger]` 属性来控制是否显示竖版分页器,例如:
```html
<nz-table [nzData]="data" [nzPageSize]="10" [nzShowSizeChanger]="false">
<!-- 这里是表格内容 -->
</nz-table>
```
希望以上方法可以解决您的问题。
相关问题
angular 去掉nz-table边框
在Angular中,如果你想要去掉Ant Design( nz-table)组件的边框,你可以通过CSS样式来实现。首先,确保你在你的样式表或者组件的style标签中导入了Ant Design的主题变量。然后,可以针对`nz-table`选择器添加以下样式:
```css
.nz-table {
border-collapse: collapse; /* 这里关闭默认的边框 */
border: none;
}
/* 如果你只想去除特定边框,比如外边框或内部行线 */
.nz-table-bordered {
border: 0 solid transparent; /* 或者设置成你喜欢的颜色,如 `border: 0 solid #ccc;` */
}
/* 如果你想移除单元格的边框 */
.nz-table-cell {
border-width: 0;
}
```
将上述代码应用到你的项目中,边框应该就会消失了。如果是在某个特定的nz-table组件上应用,记得使用`:host`伪元素来限定样式作用范围:
```css
:host(.nz-table) {
/* 样式在这里 */
}
```
编写一个Angular程序,使用ng-zorro-antd组件库中的7种组件实现相应的功能。
好的!我可以为您提供一个简单的示例程序,它使用了ng-zorro-antd组件库中的七种组件:
1. Button(按钮)
2. Input(输入框)
3. Checkbox(复选框)
4. Radio(单选框)
5. Select(下拉选择框)
6. Datepicker(日期选择器)
7. Table(表格)
以下是示例程序的代码:
app.module.ts
```typescript
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { NzButtonModule, NzInputModule, NzCheckboxModule, NzRadioModule, NzSelectModule, NzDatePickerModule, NzTableModule } from 'ng-zorro-antd';
import { AppComponent } from './app.component';
@NgModule({
imports: [ BrowserModule, FormsModule, BrowserAnimationsModule, NzButtonModule, NzInputModule, NzCheckboxModule, NzRadioModule, NzSelectModule, NzDatePickerModule, NzTableModule ],
declarations: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
```
app.component.ts
```typescript
import { Component } from '@angular/core';
interface User {
name: string;
age: number;
gender: string;
}
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
users: User[] = [
{ name: '张三', age: 20, gender: '男' },
{ name: '李四', age: 25, gender: '女' },
{ name: '王五', age: 30, gender: '男' },
];
selectedUser: User = null;
newUser: User = { name: '', age: null, gender: '' };
genderOptions = [ { label: '男', value: '男' }, { label: '女', value: '女' } ];
selectUser(user: User): void {
this.selectedUser = user;
}
addUser(): void {
this.users.push(this.newUser);
this.newUser = { name: '', age: null, gender: '' };
}
deleteUser(user: User): void {
const index = this.users.indexOf(user);
if (index >= 0) {
this.users.splice(index, 1);
this.selectedUser = null;
}
}
}
```
app.component.html
```html
<h1>用户管理</h1>
<div style="display: flex; flex-direction: row;">
<div style="flex: 1;">
<nz-table [nzData]="users" [nzFrontPagination]="false" [nzBordered]="true" [nzSize]="'middle'" (nzClick)="selectUser($event)">
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let user of users">
<td>{{ user.name }}</td>
<td>{{ user.age }}</td>
<td>{{ user.gender }}</td>
</tr>
</tbody>
</nz-table>
<div style="margin-top: 16px;">
<button nz-button [nzType]="'primary'" [disabled]="selectedUser === null" (click)="deleteUser(selectedUser)">删除</button>
</div>
</div>
<div style="flex: 1; margin-left: 16px;">
<form #userForm="ngForm">
<div>
<label>姓名:</label>
<input nz-input [(ngModel)]="newUser.name" name="name" required>
</div>
<div>
<label>年龄:</label>
<input nz-input [(ngModel)]="newUser.age" name="age" type="number" required>
</div>
<div>
<label>性别:</label>
<nz-radio-group [(ngModel)]="newUser.gender" name="gender" required>
<label nz-radio *ngFor="let option of genderOptions" [nzValue]="option.value">{{ option.label }}</label>
</nz-radio-group>
</div>
<div style="margin-top: 16px;">
<button nz-button [nzType]="'primary'" [disabled]="!userForm.valid" (click)="addUser()">添加</button>
</div>
</form>
</div>
</div>
```
这个示例程序演示了如何使用ng-zorro-antd组件库中的七种组件来实现一个简单的用户管理功能,包括显示用户列表、选择用户、添加用户和删除用户。您可以参考这个示例程序来编写自己的Angular程序,使用ng-zorro-antd组件库中的各种组件来实现您想要的功能。
阅读全文
相关推荐
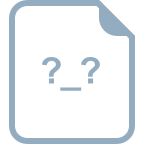
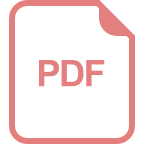
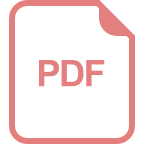
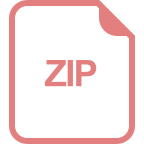
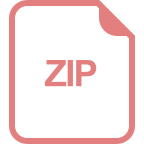
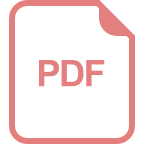
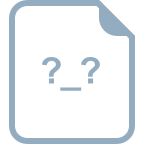
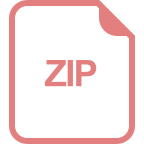
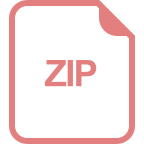
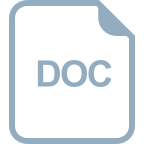
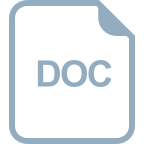
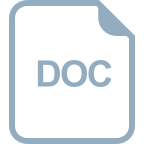


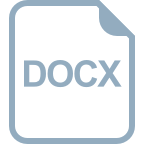
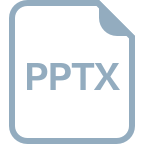