脉冲信号的均方根值的定义,请用C语言设计一个计算脉冲信号的均方根值
时间: 2024-06-09 16:07:43 浏览: 167
脉冲信号的均方根值(RMS)是指信号在一段时间内的平均功率的平方根。假设我们有一个长度为n的脉冲信号x,那么它的均方根值rms可以用下面的公式计算:
rms = sqrt((1/n) * sum(x[i]^2))
其中,sum表示求和符号,i从1到n取值。
下面是一个用C语言实现计算脉冲信号均方根值的代码示例:
```c
#include <stdio.h>
#include <math.h>
#define N 10 // 信号长度
int main() {
int x[N] = {1, -2, 3, -4, 5, -6, 7, -8, 9, -10}; // 脉冲信号
double sum = 0.0;
int i;
for (i = 0; i < N; i++) {
sum += x[i] * x[i];
}
double rms = sqrt(sum / N);
printf("脉冲信号的均方根值为:%.2f\n", rms);
return 0;
}
```
在这个示例中,我们先定义了一个长度为N的脉冲信号x,然后使用for循环遍历信号的每个元素,计算平方和sum。最后,我们将sum除以信号长度N,再开平方得到均方根值rms。最终,我们输出rms的值。
相关问题
脉冲信号的均方根定义,用STM32系列单片机对一个频率为1000hz的脉冲信号进行采集并计算该脉冲信号的有效值,请用C语言设计程序来实现以上功能
脉冲信号的均方根(RMS)定义为信号各时刻幅值平方的平均值再开根号。因此,计算脉冲信号的RMS需要先对信号进行采样,然后对每个采样点的幅值进行平方,再求平均值并开根号即可。可以使用STM32系列单片机的ADC模块对信号进行采样,并使用数学库中的函数计算均方根。
以下是示例代码:
```c
#include "stm32f4xx.h" // 根据实际使用的STM32型号修改头文件
#include <math.h> // 包含数学库函数的头文件
#define SAMPLES 100 // 采样点数
#define SAMPLING_RATE 10000 // 采样率,单位为Hz
#define SIGNAL_FREQ 1000 // 信号频率,单位为Hz
int16_t samples[SAMPLES]; // 采样数组
void ADC_Configuration(void)
{
ADC_InitTypeDef ADC_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStructure);
ADC_InitStructure.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStructure.ADC_ScanConvMode = DISABLE;
ADC_InitStructure.ADC_ContinuousConvMode = ENABLE;
ADC_InitStructure.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStructure);
ADC_Cmd(ADC1, ENABLE);
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_3Cycles);
}
int main(void)
{
float rms = 0;
int i;
ADC_Configuration();
// 等待ADC启动
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_RDY));
// 采样
for(i = 0; i < SAMPLES; i++)
{
ADC_StartOfConversion(ADC1);
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
samples[i] = ADC_GetConversionValue(ADC1);
delay_us(1000000 / SAMPLING_RATE);
}
// 计算均方根
for(i = 0; i < SAMPLES; i++)
{
rms += pow(samples[i], 2);
}
rms /= SAMPLES;
rms = sqrt(rms);
// 输出结果
printf("RMS of the pulse signal is %f\n", rms);
while (1)
{
}
}
```
以上代码使用ADC1模块对PA0引脚上的信号进行采样,并将每个采样点的值存储在采样数组中。然后,计算采样数组中每个元素的平方和,求平均值并开根号,即可得到信号的均方根。最后,将结果输出到串口中。注意,为了保证采样的准确性,需要等待ADC启动完成和采样时间。
求均方根值的C语言代码
以下是求均方根值的C语言代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
int n;
float sum = 0, mean, rms;
printf("Enter the number of values: ");
scanf("%d", &n);
float arr[n];
for (int i = 0; i < n; i++) {
printf("Enter value %d: ", i + 1);
scanf("%f", &arr[i]);
sum += arr[i];
}
mean = sum / n;
for (int i = 0; i < n; i++) {
rms += pow(arr[i] - mean, 2);
}
rms = sqrt(rms / n);
printf("The root mean square value is: %f", rms);
return 0;
}
```
代码解析:
1. 首先我们输入要求均方根值的数的个数n。
2. 然后使用一个数组arr存储输入的n个数。
3. 接着我们遍历数组,求出所有数的和。
4. 求出平均值mean。
5. 再次遍历数组,求出每个数与平均值的差的平方,然后求和。
6. 求出均方根值rms,即每个数与平均值的差的平方的平均值的平方根。
7. 最后输出均方根值rms。
注意:在计算平方和时,要将rms初始化为0,否则会出现不可预知的错误。
阅读全文
相关推荐
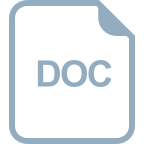
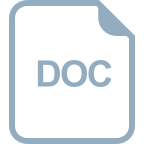
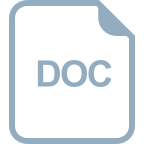
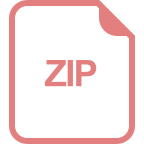







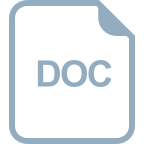
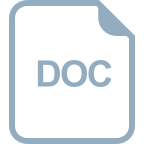
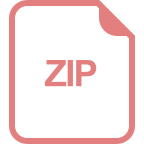
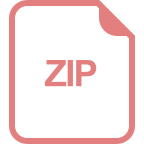
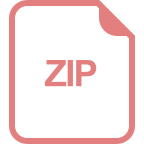
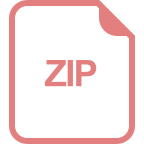